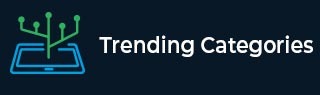
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Distribute Candies in C++
Suppose we have an array with even length, here different numbers in this array will represent different kinds of candies. Now each number means one candy of the corresponding kind. we have to distribute candies equally in number to brother and sister. We have to find the maximum number of kinds of candies the sister could receive.
So, if the input is like [1,1,2,3], then the output will be 2 as if we consider the sister has candies [2,3] and the brother has candies [1,1]. Now the sister has two different kinds of candies, the brother has only one kind of candies.
To solve this, we will follow these steps −
Define one set s
for initialize i := 0, when i < size of candies, update (increase i by 1), do −
insert candies[i] into s
return a minimum of size of s and size of candies / 2
Example
Let us see the following implementation to get a better understanding −
#include <bits/stdc++.h> using namespace std; class Solution { public: int distributeCandies(vector<int>& candies){ unordered_set<int> s; for (int i = 0; i < candies.size(); i++) s.insert(candies[i]); return min(s.size(), candies.size() / 2); } }; main(){ Solution ob; vector<int> v = {1,1,2,3}; cout << (ob.distributeCandies(v)); }
Input
{1,1,2,3}
Output
2