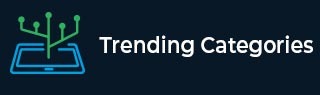
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Disable the underlying window when a popup is created in Python TKinter
We are familiar with popup and used it in many applications. Popup in a tkinter application can be created by creating an instance of Toplevel(root) window. For a particular application, we can trigger the popup on a button object.
Let us create a Python script to close the underlying or the main window after displaying the popup. We can close the main window while residing in a popup window by using the withdraw() method.
Example
Through this example, we will create a popup dialog that can be triggered after clicking a button. Once the popup will open, the parent window will close automatically.
#Import the required library from tkinter import* #Create an instance of tkinter frame win= Tk() #Define geometry of the window win.geometry("750x250") #Define a function to close the popup window def close_win(top): top.destroy() win.destroy() #Define a function to open the Popup Dialogue def popupwin(): #withdraw the main window win.withdraw() #Create a Toplevel window top= Toplevel(win) top.geometry("750x250") #Create an Entry Widget in the Toplevel window entry= Entry(top, width= 25) entry.insert(INSERT, "Enter Your Email ID") entry.pack() #Create a Button Widget in the Toplevel Window button= Button(top, text="Ok", command=lambda:close_win(top)) button.pack(pady=5, side= TOP) #Create a Label label= Label(win, text="Click the Button to Open the Popup Dialogue", font= ('Helvetica 15 bold')) label.pack(pady=20) #Create a Button button= Button(win, text= "Click Me!", command= popupwin, font= ('Helvetica 14 bold')) button.pack(pady=20) win.mainloop()
Output
When we execute the above code snippet, it will display a window.
Now click the "Click Me" button. It will open a Popup window and close the parent window.