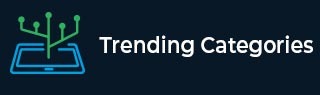
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Differences between Flatten() and Ravel() in Numpy
There are numerous ways to create a numpy array. Numpy provides two different kinds of ways to convert a ndarray to 1Darray: that is using the flatten() method and the other using the ravel() method.
Example
#Import required library, numpy import numpy as np #create an array from a list arr = np.array( [ (2, 7, 3, 4), (5, 6, 9, 1)]) #flatten_output print(arr.flatten()) #ravel_output print(arr.ravel())
Output
[2 7 3 4 5 6 9 1] [2 7 3 4 5 6 9 1]
Now above we can see that both functions return the same list, so the question arises, why two methods for the same task?
Below are the key differences between flatten() and ravel() method.
arr.ravel()
Return the only reference of original array
On modifying the above array(arr), we can see that the value of the original array also changes.
Because ravel method does occupy any memory, ravel is faster than flatten()
Ravel is a library level function
arr.flatten()
Returns an original copy of array(arr).
On modifying the above array(arr), the value original array will not change.
Because flatten() occupies memory, flatten() is little-bit slower than ravel()
It’s a method of a ndarray object.
Example
#Import required library, numpy import numpy as np # Create a numpy array, arr arr = np.array([(1,2,3,4),(3,1,4,2)]) # Let's print the array arr print ("Original array:\n ", arr) #print(arr) # To check the dimension of array (dimension =2) and type is numpy.ndarray print ("Dimension of original array: %d \n Type of original array: %s" % (arr.ndim,type(arr))) print("\nOutput from ravel method: \n") # Convert nd array to 1D array b_arr = arr.ravel() # Ravel only passes a view of original array to array 'b_arr' print(b_arr) b_arr[0]=1000 print(b_arr) # Note here that value of original array 'arr' at also arr[0][0] becomes 1000 print(arr) # Just to check the dimension i.e. 1 and type is same numpy.ndarray print ("Dimension of array: %d \n Type of array: %s" % (b_arr.ndim,type(b_arr))) print("\nOutput from flatten method: \n") # Convert nd array to 1D array c_arr = arr.flatten() # Flatten passes copy of original array to 'c_arr' print(c_arr) c_arr[0]=0 print(c_arr) # Note that by changing value of c_arr there is no affect on value of original array 'arr' print(arr) print ("Dimension of array->%d \n Type of array->%s" % (c_arr.ndim,type(c_arr)))
Output
Original array: [[1 2 3 4] [3 1 4 2]] Dimension of original array: 2 Type of original array: <class 'numpy.ndarray'> Output from ravel method: [1 2 3 4 3 1 4 2] [1000 2 3 4 3 1 4 2] [[1000 2 3 4] [ 3 1 4 2]] Dimension of array: 1 Type of array: <class 'numpy.ndarray'> Output from flatten method: [1000 2 3 4 3 1 4 2] [0 2 3 4 3 1 4 2] [[1000 2 3 4] [ 3 1 4 2]] Dimension of array->1 Type of array-><class 'numpy.ndarray'>