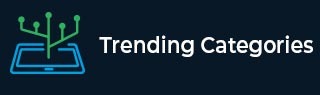
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Difference between while(1) and while(0) in C language
As we know that in C language 'while' keyword is used to define a loop which works on the condition passed as argument to the loop. Now as condition can have two values either true or false so the code inside while block will be executed repeatedly if condition is true and if condition is false the code will not be executed.
Now passing the argument to the while loop we can distinguish between while(1) and while(0) as while(1) is the loop where the condition is always treated as true and so the code inside the block start executing repeatedly. Additionally, we can state that it is not 1 which gets passed to the loop and makes the condition true but if any non-zero integer made to pass in while loop then it would be treated as true condition and hence code starts executing.
On other hand while(0) is the loop where the condition is always treated as false and so the code inside the block never gets to start executing. Additionally, we can state that it is only 0 which get passed to the loop and makes the condition false so if any other non-zero integer it may be negative also made to pass in while loop then it would be treated as true condition and hence code starts executing.
Point discussed above could be demonstrated by the help of below illustrated example.
Example
Example of while(1)
#include using namespace std; main(){ int i = 0; cout << "Loop get started"; while(1){ cout << "The value of i: "; if(i == 10){ //when i is 10, then come out from loop break; } } cout << "Loop get ended" ; }
Output
Loop get started The value of i: 1 The value of i: 2 The value of i: 3 The value of i: 4 The value of i: 5 The value of i: 6 The value of i: 7 The value of i: 8 The value of i: 9 The value of i: 10 Loop gets ended
Example
Example of while(0)
#include using namespace std; main(){ int i = 0; cout << "Loop get started"; while(0){ cout << "The value of i: "; if(i == 10){ //when i is 10, then come out from loop break; } } cout << "Loop get ended" ; }
Output
Loop get started Loop get ended