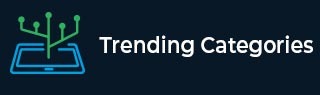
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Difference between test () and exec () methods in Javascript
The RegExp.prototype.test() and RegExp.prototype.exec() methods are the methods in JavaScript used to handle regular expressions. In addition to these two JavaScript includes a number of built-in methods for manipulating and processing text using regular expressions.
What are regular expressions?
Regular expressions are patterns that are used to search for character combinations in a string. Regular expressions are treated as objects in JavaScript and are denoted by either "regex" or "RegExp."
The exec() method
The exec() method makes a search for the specified match of the string in the given string. If the match of the string is present in the given string it returns an array and if the match is not found in the given string, then it returns a null value. In other words, The exec() method takes a string as the parameter. This string is which is to be checked for match in the given string.
Syntax
This is the syntax of the exec() method of regular expressions in JavaScript −
regularExpressionObj.exec(string)
Example
Following is an example of the exec() method in JavaScript. In here we are searching for a particular word in the given string.
<!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>exec() - Regular Expression in JavaScript</title> </head> <body> <p>click to get exec() method output</p> <button onclick="findMatch()">Search</button> <p id="tutorial"></p> <script> function findMatch() { var txt ="Learning regular expressions in JavaScript"; var search1 = new RegExp("JavaScript"); var search2 = new RegExp("Abdul") var res1 = search1.exec(txt); var res2 = search2.exec(txt); document.getElementById("tutorial").innerHTML ="Given string is:"+txt +"<br>"+ "Specific word to match are:"+search1+" "+search2+"<br>"+"Result of two search keys: "+res1+" "+res2 } </script> </body> </html>
The test() method
The test() method makes a search for the specified match of the string in the given string. The difference in between exec() and test() method is that the exec() method will return the specified match if present or null if not present in the given string whereas the test() method returns a Boolean result i.e., true if the specified match is present or else returns false if it is not present.
Syntax
This is the syntax of the test() method in JavaScript −
regularExpressionObj.test(string)
This also takes the given input string as the parameter and returns the Boolean result.
Example
This programs also searches for a given word in a string but in here we are using the test() method.
<!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>test() - Regular Expression in JavaScript</title> </head> <body> <p>click to get exec() method output</p> <button onclick="findMatch()">Search</button> <p id="tutorial"></p> <script> function findMatch() { var txt ="Learning regular expressions in JavaScript"; var search1 = new RegExp("JavaScript"); var search2 = new RegExp("Abdul") var res1 = search1.test(txt); var res2 = search2.test(txt); document.getElementById("tutorial").innerHTML ="Given string is:"+txt+"<br>"+ "Specific word to match are:"+search1+" "+search2+"<br>"+"Result of two search keys: "+res1+" "+res2 } </script> </body> </html>
Difference Between search() & test()
The test() method verifies for matches and returns a boolean value whereas the exec() method captures groups and matches the regex to the input. If you only need to test an input string to match a regular expression, RegExp.test() is most appropriate. It will give you a boolean value which makes it ideal for conditions.
The RegExp.exec() method gives you an array-like return value with all capture groups and matched indexes. Therefore, it is useful when you need to work with the captured groups or indexes after the match.
Example
In the following example we are executing the same regular expression using both the methods. In here you can observe that the test() method returned a boolean value and the exec() method returned an array.
<!DOCTYPE html> <html> <body> <script> var regex = RegExp("^([a-z]+) ([A-Z]+)$"); document.write("Result of the exec method: "+regex.exec("hello WORLD")) document.write("<br> Result of the test method: "+regex.test("hello WORLD")) </script> </body> </html>
Output
On executing the above program, you will get the following output.
Result of the exec method: hello WORLD,hello,WORLD Result of the test method: true
Note that the first index in the array returned by exec is the complete matched string. The following indices are the individual groups captured by the regex.