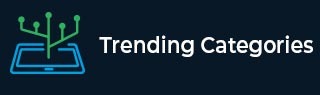
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Difference between Procedural Programming Languages and Functional Programming Languages
Procedural programming is a type of programming in which different functions or routines are created which consists of a sequence of instructions. Functional programming is used to organize the functions. Each function has to be set in such a way that they can perform the required task. We will discuss the difference between procedural programming languages and functional programming languages.
Procedural Programming Languages
Procedural programming languages have functions which you have to define. These functions need to have a set of instructions which should be executed when the function is called. You have to think about each instruction that you have to execute. Proper organization of instructions is needed so that they can be executed successfully.
An example of a procedure is given below −
#include <stdio.h> void poo() { int a = 1; int b = 2; int c = a + b; printf(“Result: %d/n”, c); } int main() ( poo(); }
In this example, you can see the steps which are written in a proper sequence. A linear, top-down approach is followed in the procedural programming languages in which each function consists of a series of code. Real-world resemblance to different objects cannot be created in these languages. This is a feature of object-oriented languages.
Procedural Programming Languages Features
Procedural programming languages have many features which are discussed below −
Pre-defined Functions
These are the functions that are already available in the libraries of the programming languages. You just have to use the library and call the function. Usually, high-level programming languages have the features of libraries and pre-defined functions. In order to print something in Java, you have to use system.out.println(). This function is used to print anything on the command line or console.
Local Variable
A local variable is a variable that is defined inside a function and can be used in that function only. It cannot be called by other functions. This helps the function to use the variable and the rest of the code is not disturbed.
An example is given below −
#include <stdio.h> void sum() { int a = 4; int b = 5; int c = a + b; printf("Result: %d
", c); } int main() { sum(); }
In this example, variables a, b, and c are local to the function sum() and cannot be called by other functions.
Global Variable
A global variable is a variable which is defined outside the functions and can be used by any function. You cannot define a variable with the same name as a global variable in any function. These variables can be accessed from any function.
Here is an example of a global variable −
#include <stdio.h> int a; void sum() { a=1; int b = 2; int c = a + b; printf("Result: %d
", c); } void difference() { a = 5; int b = 4; int c = a - b; printf("Result: %d
", c); } int main() { sum(); difference(); }
In this example, variable a is global and it is accessed in the function sum() and difference(). This variable can be accessed from any function. Variables b and c are local to sum() and difference() functions so they cannot be used by other functions.
Modularity
Modularity is a process in software development in which the functionality of the software is divided into different modules. Each module has to perform its task along with other modules.
Advantages of Procedural Programming Languages
Procedural programming languages have many advantages and we will enlist some of them here.
- It is a good option if you are writing general-purpose programs
- It is a simple way of writing code and is a good option for beginners who want to learn programming
- The flow of the program can be tracked easily and this helps in removing the errors if any
Disadvantages of Procedural Programming Languages
Procedural programming languages have a few disadvantages and they are listed below −
- The code of the procedural programming languages cannot be reused
- The code is not secured as data is visible across the whole program
Examples of Procedural Programming Languages
Some of the procedural programming languages are as follows −
Functional Programming Languages
Functional programming is a type of programming in which the code is organized on the basis of functions. A task is defined in each function along with parameters. Necessary data is passed within the parameters to get the results. Functional programming is based on pure functions.
Pure Functions
A pure function is responsible for providing the same output on the basis of the given inputs. For example, if we use math.cos(x) function, the result will depend on the value of x. The math.cos(x) function does not disturb the rest of the code. Pure functions have many features listed below −
- The output of the pure functions is the same and depends on the given input
- A pure function has referential transparency when a function is called and the value is replaced
- The input arguments in the pure functions do not modify anything given in the whole program
- Only parameters given in the function are used and they work only on the given variables
An example of a pure function is given below −
#include <stdio.h> int sum(a, b) { return a+b; } int main() { int var1 = 1; int var2 = 2; int var_add = sum(var1, var2); printf("Result: %d
", var_add); }
Concepts of Functional Programming
Here we will discuss different concepts of functional programming −
Immutable Variable
An immutable variable can never be changed after being initialized while a mutable variable can be changed by giving different values. In functional programming, only immutable variables can be used. If you have to change the value, the immutable variable should be initialized in a new variable.
Shared State is avoided
Variables may also be defined as having a shared scope. The value of such variables can be modified from anywhere. Tracking such variables is very difficult. So shared state of variables is not used in functional programming.
Recursion
Recursion is a concept which is used as an alternative for iteration which is done with the help of while() and for() loops. Each function call is repeated until it meets the base.
Advantages of Functional Programming Languages
Functional programming languages have many advantages and they are listed below −
- The same output is delivered by the pure functions and the rest of the program is not disturbed
- Functional programming is transparent as the parameters can be passed explicitly to return a value
- Developers can debug the whole program easily
Disadvantages of Functional Programming
Functional programming has a few disadvantages which are listed below −
- The usage of recursion for each iteration can be problematic
- The immutability of variables reduces the performance of the code
Examples of Functional Programming Languages
Difference between Procedural Programming Languages and Functional Programming Languages
Here we will discuss the difference between procedural programming languages and functional programming languages and they are given in the table below −
Functional Programming Languages | Procedural Programming Languages |
---|---|
Data and functions do not have any difference. The output depends on the functions. | Data and functions have differences and output depends on the operation of the functions. |
Parallel and concurrent programming is done through the functional programming languages. | Procedural programming languages are used for general-purpose programming |
Data available in the functional programming is immutable | Data can be accessed from anywhere at any time |
Code is organized with the help of modules and functions | Code is organized through modules and procedures |
Testing is easy | Testing is difficult |
Its performance is slow due to the usage of a large number of variables | Its performance is fast |
Conclusion
Procedural and functional programming languages have their own advantages and disadvantages. They differ from each other on the basis of variable declaration, functions, shared variables, etc. Both of them are being used in the modern programming.