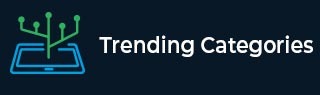
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Difference Between Del and Remove() on Lists in Python?
Let us understand what is Del and Remove() in a Python List before moving towards the difference.
Del Keyword in Python List
The del keyword in Python is used to remove an element or multiple elements from a List. We can also remove all the elements i.e. delete the entire list.
Example
Delete an element from a Python List using the del keyword
#Create a List myList = ["Toyota", "Benz", "Audi", "Bentley"] print("List = ",myList) # Delete a single element del myList[2] print("Updated List = \n",myList)
Output
List = ['Toyota', 'Benz', 'Audi', 'Bentley'] Updated List = ['Toyota', 'Benz', 'Bentley']
Example
Delete multiple elements from a Python List using the del keyword
# Create a List myList = ["Toyota", "Benz", "Audi", "Bentley", "Hyundai", "Honda", "Tata"] print("List = ",myList) # Delete multiple element del myList[2:5] print("Updated List = \n",myList)
Output
List = ['Toyota', 'Benz', 'Audi', 'Bentley', 'Hyundai', 'Honda', 'Tata'] Updated List = ['Toyota', 'Benz', 'Honda', 'Tata']
Example
Delete all the elements from a Python List using the del keyword
# Create a List myList = ["Toyota", "Benz", "Audi", "Bentley"] print("List = ",myList) # Deletes the entire List del myList # The above deleted the List completely and all its elements
Output
List = ['Toyota', 'Benz', 'Audi', 'Bentley']
Remove() method in Python List
The remove() built-in method in Python is used to remove elements from a List.
Example
Remove an element from a Python using the remove() method
# Create a List myList = ["Toyota", "Benz", "Audi", "Bentley"] print("List = ",myList) # Remove a single element myList.remove("Benz") # Display the updated List print("Updated List = \n",myList)
Output
List = ['Toyota', 'Benz', 'Audi', 'Bentley'] Updated List = ['Toyota', 'Audi', 'Bentley']
Del vs Remove()
Let us now see the difference between del and remove() in Python −
del in Python | remove() |
---|---|
The del is a keyword in Python. | The remove(0) is a built-in method in Python. |
An indexError is thrown if the index doesn’t exist in the Python list. | The valueError is thrown if the value doesn’t exist in the Python list. |
The del works on index. | The remove() works on value. |
The del deletes an element at a specified index number. | It removes the first matching value from the Python List. |
The del is simple deletion. | The remove() searches the list to find the item. |
Advertisements