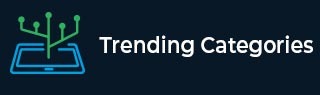
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Difference between ArrayList and HashSet in Java
HashSet and ArrayList both are some of the most important classes of the Java Collection framework.
The following are the important differences between ArrayList and HashSet.
Sr. No. | Key | ArrayList | HashSet |
---|---|---|---|
1 | Implementation | ArrayList is the implementation of the list interface. | HashSet on the other hand is the implementation of a set interface. |
2 | Internal implementation | ArrayList internally implements array for its implementation. | HashSet internally uses Hashmap for its implementation. |
3 | Order of elements | ArrayList maintains the insertion order i.e order of the object in which they are inserted. | HashSet is an unordered collection and doesn't maintain any order. |
4 | Duplicates | ArrayList allows duplicate values in its collection. | On other hand duplicate elements are not allowed in Hashset. |
5 | Index performance | ArrayList uses index for its performance i.e its index based one can retrieve object by calling get(index) or remove objects by calling remove(index) | HashSet is completely based on object also it doesn't provide get() method. |
6 | Null Allowed | Any number of null value can be inserted in arraylist without any restriction. | On other hand Hashset allows only one null value in its collection,after which no null value is allowed to be added. |
Example of ArrayList vs Hashset
JavaTester.java
import java.io.*; import java.util.*; public class JavaTester { public static void main(String[] args) throws IOException{ int n = 5; List<Integer> al = new ArrayList<>(n); for (int i = 1; i <= n; i++) { al.add(i); } System.out.println(al); al.remove(3); System.out.println(al); for (int i = 0; i < al.size(); i++) { System.out.print(al.get(i) + " "); } } }
Output
[1, 2, 3, 4, 5] [1, 2, 3, 5] 1 2 3 5
Example
JavaTester.java
import java.util.HashSet; import java.util.Set; public class JavaTester { public static void main(String[] args){ Set<Integer> hs = new HashSet<>(); hs.add(1); hs.add(2); hs.add(3); hs.add(4); hs.add(4); for (Integer temp : hs) { System.out.print(temp + " "); } } }
Output
1 2 3 4
Advertisements