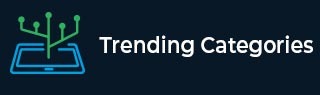
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Dictionary to list of tuple conversion in Python
ging the collection type from one type to another is a very frequent need in python. In this article we will see how we create a tuple from the key value pairs that are present in a dictionary. Each of the key value pair becomes a tuple. So the final list is a list whose elements are tuples.
With items()
We sue the items method of the dictionary which allows us to iterate through each of the key value pairs. Then we use a for loop to pack those values in to a tuple. We put all these tuples to a final list.
Example
dictA = {'Mon': '2 pm', 'Tue': '1 pm', 'Fri': '3 pm'} # Using items() res = [(k, v) for k, v in dictA.items()] # Result print(res)
Output
Running the above code gives us the following result −
[('Mon', '2 pm'), ('Tue', '1 pm'), ('Fri', '3 pm')]
With zip
Another approach is to use the zip function. The zip function will pair the keys and values as tuples and then we convert the entire result to a list by applying list function.
Example
dictA = {'Mon': '2 pm', 'Tue': '1 pm', 'Fri': '3 pm'} # Using items() res = list(zip(dictA.keys(), dictA.values())) # Result print(res)
Output
Running the above code gives us the following result −
[('Mon', '2 pm'), ('Tue', '1 pm'), ('Fri', '3 pm')]
With append
The append() can append the result into a list after fetching the pair of values to create a tuple. We iterate through a for loop to get the final result.
Example
dictA = {'Mon': '2 pm', 'Tue': '1 pm', 'Fri': '3 pm'} # Initialize empty list res=[] # Append to res for i in dictA: tpl = (i, dictA[i]) res.append(tpl) # Result print(res)
Output
Running the above code gives us the following result −
[('Mon', '2 pm'), ('Tue', '1 pm'), ('Fri', '3 pm')]