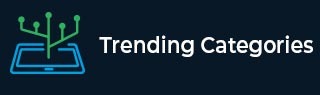
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Detect Capital in a given string using C++
Let's suppose we have a string ‘str’ which consists of some character in it. The task is to check whether the given string has all its characters capitalized or not and return True or False respectively. For example,
Input-1 −
str = “INDIA”
Output −
True
Explanation − Since all the character of the input string is capital, we will return true in this case.
Input-2 −
str = “Programmer”
Output −
False
Explanation − Since all the characters of the input string are not in the capital except the first letter, we will return false in this case.
The approach used to solve this problem
In the given string, we have to check whether all the characters of the input string are capitalized or not. Thus, we will iterate over each of the characters of the string and will check if it is greater than ‘A’ and less than ‘Z’.
If the condition is true, then we will increase the counter. Then, we will move ahead and again check if the size of the counter is the same as the length of the string, then return true, otherwise false.
Take input of a string ‘str’
A Boolean function checkCapital(string s) takes a string as input and returns true if all the characters of the string are capitalized.
Take a counter variable and initialize it as zero.
Iterate over the string and check if the current character falls under the range of ‘A’ to ‘Z’ if the condition is true then increment the counter variable.
Now the check counter is the same as the size of the string and returns True/False accordingly.
If the counter is 0 or the string is having one character which is capital, then return true.
Example
#include<bits/stdc++.h> using namespace std; bool checkCapital(string s){ int counter=0; int loc=i; for(int i=0;i<s.size();i++){ if(s[i]>='A' && s[i]<= 'Z'){ counter++; loc=i; } } if(counter==s.size() || counter==0 ||(counter==1 && loc==0)) return true; return false; } int main(){ string str= "INDIA"; bool ans= checkCapital(str); if(ans){ cout<<"True"<<endl; } else { cout<<"False"<<endl; } return 0; }
Output
Running the above code will generate the output as,
True
Since the input string ‘INDIA’ contains all the character capital, we get the output as “True”.