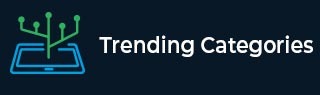
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Destructively Sum all the digits of a number in JavaScript
Our main task is to write the function to destructively sum all the digits of a number with the help of Javascript. So for doing this task we will use a while loop and a function to get the desired output.
Understanding the problem statement
The problem is to create a function to calculate the sum of all the digits in the given number. Means we have given a number and we have to calculate the sum of all the digits in a destructive manner as the original number is modified during the process.
For example let's say we have a number 123. So we need to calculate the sum of the given digits in this number 1 + 2 + 3 = 6.
So our aim is to write an algorithm which can compute the sum by fulfilling the above requirements.
Logic for the above problem
To solve the problem we will use a while loop to continuously extract the rightmost digit of the input number with the help of a modulus operator and add it to a running total number. Then we will remove the rightmost digit by dividing the number by 10 and round it down with the help of Math.floor method. We will repeat this process until all the digits of the given number have been extracted and added to the total of the number. So at that point of time we can return the final sum.
Algorithm
Step 1 − Create a function to calculate the digit sum of the given number and give it a name as getDigitSum and pass an argument called num.
Step 2 − Define a variable to store the sum of the given digits of the number and give it a name as sum.
Step 3 − Use a while loop to verify the condition and process accordingly. And inside the loop check that the given number is greater than zero.
Step 4 − We will calculate the sum and num with the help of modulus operator and Math.floor methods.
Step 5 − Outside the loop we will return the calculated sum of the digits.
Code for the algorithm
//function to calculate the sum of all the digits function getDigitSum(num) { let sum = 0; while (num > 0) { sum += num % 10; num = Math.floor(num / 10); } return sum; } const num = 123456; const digitSum = getDigitSum(num); console.log(digitSum);
Complexity
The time taken by the produced function is O(log n) in which n is the value of the given number. This is because the number of iterations in the while loop is equally proportional to the number of digits in number. And the space complexity of the algorithm is O(1) which is constant, because it only uses a constant amount of additional space to store the sum.
Conclusion
The above function provides an efficient solution for finding the sum of all the digits of a number with the usage of Javascript programming language. In the code we have repeated the process until all the digits have been extracted and added to the current total.