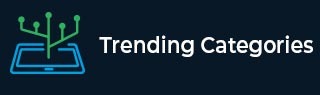
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Density of Binary Tree in One Traversal in C++?
The density of a binary tree is calculated by dividing its size by its height.Binary tree density = size/height.
Let us first define the struct that would represent a tree node that contains the data and its left and right node child. If this is the first node to be created then it’s a root node otherwise a child node.
struct Node { int data; struct Node *leftChild, *rightChild; };
Next we create our createNode(int data) function that takes an int value and assign it to the data member of the node. The function returns the pointer to the created struct Node. Also, the left and right child of the newly created node are set to null.
Node* createNode(int data){ Node* node = new Node; node->data = data; node->leftChild = node->rightChild = NULL; return node; }
Our treeDensity(Node *root) function takes the root node and checks if its null or not. It then declares the size variable and set it to 0. The return value of heightAndSize(root, size) function is assigned to the height variable. The float division performed between height and size is then returned.
float treeDensity(Node* root){ if (root == NULL) return 0; int size = 0; int height = heightAndSize(root, size); return (float)size/height; }
Next, the heightAndSize(Node* node, int &size) takes the root node and the reference to the size variable. If the root node is null then 0 is returned. The height of each subtree is calculated recursively and the size is increased on each recursion. We then return the larger of the left or right subtree.
int heightAndSize(Node* node, int &size){ if (node==NULL) return 0; int left = heightAndSize(node->leftChild, size); int right = heightAndSize(node->rightChild, size); size++; return (left > right) ? ++left : ++right; }
Example
Let us look at the following implementation of finding the binary tree density in one traversal −
#include<iostream> using namespace std; struct Node{ int data; Node *leftChild, *rightChild; }; Node* createNode(int data){ Node* node = new Node; node->data = data; node->leftChild = node->rightChild = NULL; return node; } int heightAndSize(Node* node, int &size){ if (node==NULL) return 0; int left = heightAndSize(node->leftChild, size); int right = heightAndSize(node->rightChild, size); size++; return (left > right) ? ++left : ++right; } float treeDensity(Node* root){ if (root == NULL) return 0; int size = 0; int height = heightAndSize(root, size); return (float)size/height; } int main(){ Node* root = createNode(7); root->leftChild = createNode(9); root->rightChild = createNode(11); cout<< "The density of the above given binary tree is "<<treeDensity(root); return 0; }
Output
The above code will produce the following output −
The density of the above given binary tree is 1.5