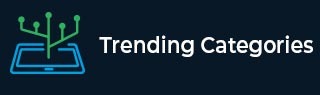
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
DateTimeOffset.AddDays() Method in C#
The DateTimeOffset.AddDays() method in C# is used to return a new DateTimeOffset object that adds a specified number of whole and fractional days to the value of this instance.
Syntax
Following is the syntax −
public DateTimeOffset AddDays (double val);
Above, Val is the number of days to be added. To subtract, include a negative value.
Example
Let us now see an example to implement the DateTimeOffset.AddDays() method −
using System; public class Demo { public static void Main() { DateTimeOffset dateTimeOffset = new DateTimeOffset(2019, 10, 10, 9, 15, 0, new TimeSpan(-5, 0, 0)); DateTimeOffset res = dateTimeOffset.AddDays(20); Console.WriteLine("DateTimeOffset = {0}", res); } }
Output
This will produce the following output −
DateTimeOffset = 10/30/2019 9:15:00 AM -05:00
Example
Let us now see another example to implement the DateTimeOffset.AddDays() method −
using System; public class Demo { public static void Main() { DateTimeOffset dateTimeOffset = new DateTimeOffset(2019, 10, 10, 9, 15, 0, new TimeSpan(-5, 0, 0)); DateTimeOffset res = dateTimeOffset.AddDays(-5); Console.WriteLine("DateTimeOffset (after subtracting days) = {0}", res); } }
Output
This will produce the following output −
DateTimeOffset (after subtracting days) = 10/5/2019 9:15:00 AM -05:00
Advertisements