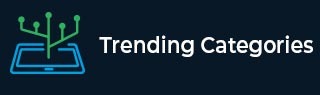
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
DateTime.SpecifyKind() Method in C#
The DateTime.SpecifyKind() method in C# is used to create a new DateTime object that has the same number of ticks as the specified DateTime but is designated as either local time, Coordinated Universal Time (UTC), or neither, as indicated by the specified DateTimeKind value.
Syntax
Following is the syntax −
public static DateTime SpecifyKind (DateTime d, DateTimeKind kind);
Above, the parameter d is the DateTime, whereas kind is One of the enumeration values that indicates whether the new object represents local time, UTC, or neither.
Example
Let us now see an example to implement the DateTime.SpecifyKind() method −
using System; using System.Globalization; public class Demo { public static void Main() { DateTime d = new DateTime(2019, 10, 11, 11, 10, 42); DateTime res = DateTime.SpecifyKind(d, DateTimeKind.Local); Console.WriteLine("Kind = " + res.Kind); } }
Output
This will produce the following output −
Kind = Local
Example
Let us now see another example to implement the DateTime.SpecifyKind() method −
using System; public class Demo { public static void Main() { DateTime d = new DateTime(2019, 11, 25, 10, 20, 35); DateTime res = DateTime.SpecifyKind(d, DateTimeKind.Utc); Console.WriteLine("Kind = " + res.Kind); } }
Output
This will produce the following output −
Kind = Utc
Advertisements