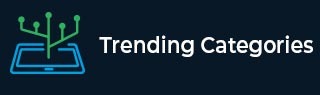
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
DateTime.AddSeconds() Method in C#
The DateTime.AddSeconds() method in C# is used to add the specified number of seconds to the value of this instance. This returns a new DateTime.
Syntax
Following is the syntax −
public DateTime AddSeconds (double sec);
Here, sec is the seconds to be added. If you want to subtract seconds, then set a negative value.
Example
Let us now see an example to implement the DateTime.AddSeconds() method −
using System; public class Demo { public static void Main(){ DateTime d1 = new DateTime(2019, 10, 25, 6, 20, 40); DateTime d2 = d1.AddSeconds(25); System.Console.WriteLine("Initial DateTime = {0:dd} {0:y}, {0:hh}:{0:mm}:{0:ss} ", d1); System.Console.WriteLine("
New DateTime (After adding seconds) = {0:dd} {0:y}, {0:hh}:{0:mm}:{0:ss} ", d2); } }
Output
This will produce the following output −
Initial DateTime = 25 October 2019, 06:20:40 New DateTime (After adding seconds) = 25 October 2019, 06:21:05
Example
Let us now see another example to implement the DateTime.AddSeconds() method −
using System; public class Demo { public static void Main(){ DateTime d1 = new DateTime(2019, 10, 25, 6, 20, 40); DateTime d2 = d1.AddSeconds(-10); System.Console.WriteLine("Initial DateTime = {0:dd} {0:y}, {0:hh}:{0:mm}:{0:ss} ", d1); System.Console.WriteLine("
New DateTime (After subtracting seconds) = {0:dd} {0:y}, {0:hh}:{0:mm}:{0:ss} ", d2); } }
Output
This will produce the following output −
Initial DateTime = 25 October 2019, 06:20:40 New DateTime (After subtracting seconds) = 25 October 2019, 06:20:30
Advertisements