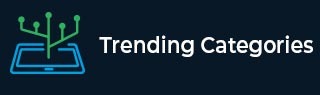
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Daily Temperatures in C++
Let us suppose we have an array of positive temperatures which represent the temperatures T. The task is to calculate how many days are there for the next warmer temperature in the given list.
For example
Input-1: T = [ 73, 74, 75, 71, 69, 72, 76, 73]
Output: [1, 1, 4, 2, 1 ,1 ,0 ,0]
Explanation: In the given list of temperatures [73, 74, 75, 71, 69, 72, 76, 73], the next greater temperature is at Day 1. Similarly, Day 6 is the warmest in all the temperatures, thus the output will be [ 1, 1, 4, 2, 1, 1, 0, 0].
Approach to Solve this Problem
We have a list of temperatures and we have to calculate the number of days till the next warmer day from the given temperatures.
To solve this problem, we can use a Stack. Initially the stack is empty, and we will check if the top of the stack is empty, then push the temperature. Next, if the temperature on the top of the stack is lesser than the next available temperature, it means that it is colder, and we will pop it from the stack.
We will check again if the top of the stack has the temperature greater or warmer, then calculate the day by subtracting the days from it and store the index in the result.
- Take input of temperature data.
- A integer function dailyTemperature(int *T) takes the temperature array as the input and returns the list of the next warmest temperature.
- Iterate over the temperature array.
- Create a result vector or array to return the list of all the temperatures.
- Create an empty stack and push the temperature while checking if top()>T[i], then push it into the stack and calculate the days.
- Store the result and return it.
Example
#include<bits/stdc++.h> using namespace std; void dailyTemp(int * T, int n) { stack < int > s; int ans[n]; memset(ans, 0, sizeof(ans)); for (int i = 0; i < n; i++) { while (!s.empty() && T[s.top()] < T[i]) { int j = s.top(); s.pop(); ans[j] = i - j; } s.push(i); } for (int i = 0; i < n; i++) { cout << ans[i] << " " << ; } } int main() { int n = 8; int T[8] = {73, 74, 75, 71, 69, 72, 76, 73}; dailyTemp(T, n); return 0; }
Output
Running the above code will generate the output as,
1 1 4 2 1 1 0 0
The next warmer temperature in the given temperature is at Day 4.