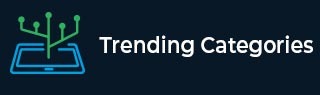
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Custom Jumble Word Game
In this article, we will explore the concept of creating a custom Jumble Word Game in C++. Word puzzles are not only entertaining but also a great way to improve vocabulary and cognitive skills. We will walk you through the process of designing and implementing the game using C++ and provide a test case example to illustrate how the game works.
Custom Jumble Word Game
The objective of the Jumble Word Game is to unscramble a given set of letters to form a valid word. Players are given a jumbled word, and they have to rearrange the letters to form the original word. To make the game more engaging, you can add features like hints, levels, or time constraints.
C++ Implementation
Example
Here are the programs that implements the above strategy: −
#include <stdio.h> #include <string.h> #include <time.h> #include <stdlib.h> // Function to jumble the word void jumbleWord(char *word) { int length = strlen(word); for (int i = 0; i < length - 1; i++) { int j = i + rand() % (length - i); char temp = word[i]; word[i] = word[j]; word[j] = temp; } } int main() { srand((unsigned int)time(NULL)); char originalWord[] = "programming"; char jumbledWord[12]; // One extra for the null terminator '\0' strcpy(jumbledWord, originalWord); jumbleWord(jumbledWord); // Simulate user actions char firstUserGuess[] = "prominggam"; char userHintRequest[] = "hint"; char secondUserGuess[] = "programming"; printf("Welcome to the Jumble Word Game!\n"); printf("Unscramble the letters to form a word.\n"); printf("Enter 'hint' for a hint.\n"); printf("Enter 'quit' to quit the game.\n"); printf("The jumble word is: %s\n", jumbledWord); // Simulate the first user guess printf("User's first guess: %s\n", firstUserGuess); if (strcmp(firstUserGuess, originalWord) == 0) { printf("Congratulations! You have successfully unscrambled the word!\n"); } else { printf("Incorrect guess. Please try again!\n"); } // Simulate user asking for a hint if (strcmp(userHintRequest, "hint") == 0) { printf("The first letter of the word is: %c\n", originalWord[0]); } // Simulate the second user guess printf("User's second guess: %s\n", secondUserGuess); if (strcmp(secondUserGuess, originalWord) == 0) { printf("Congratulations! You have successfully unscrambled the word!\n"); } else { printf("Incorrect guess. Please try again!\n"); } return 0; }
Output
Welcome to the Jumble Word Game! Unscramble the letters to form a word. Enter 'hint' for a hint. Enter 'quit' to quit the game. The jumble word is: pmiarnogrmg User's first guess: prominggam Incorrect guess. Please try again! The first letter of the word is: p User's second guess: programming Congratulations! You have successfully unscrambled the word!
#include <iostream> #include <string> #include <ctime> #include <cstdlib> #include <algorithm> std::string jumbleWord(const std::string &word) { std::string jumbledWord = word; std::random_shuffle(jumbledWord.begin(), jumbledWord.end()); return jumbledWord; } int main() { srand(static_cast<unsigned int>(time(0))); std::string originalWord = "programming"; std::string jumbledWord = jumbleWord(originalWord); // Simulate user actions std::string firstUserGuess = "prominggam"; std::string userHintRequest = "hint"; std::string secondUserGuess = "programming"; std::cout << "Welcome to the Jumble Word Game!" << std::endl; std::cout << "Unscramble the letters to form a word." << std::endl; std::cout << "Enter 'hint' for a hint." << std::endl; std::cout << "Enter 'quit' to quit the game." << std::endl; std::cout << "The jumble word is: " << jumbledWord << std::endl; // Simulate the first user guess std::cout << "User's first guess: " << firstUserGuess << std::endl; if (firstUserGuess == originalWord) { std::cout << "Congratulations! You have successfully unscrambled the word!" << std::endl; } else { std::cout << "Incorrect guess. Please try again!" << std::endl; } // Simulate user asking for a hint if (userHintRequest == "hint") { std::cout << "The first letter of the word is: " << originalWord[0] << std::endl; } // Simulate the second user guess std::cout << "User's second guess: " << secondUserGuess << std::endl; if (secondUserGuess == originalWord) { std::cout << "Congratulations! You have successfully unscrambled the word!" << std::endl; } else { std::cout << "Incorrect guess. Please try again!" << std::endl; } return 0; }
Output
Welcome to the Jumble Word Game! Unscramble the letters to form a word. Enter 'hint' for a hint. Enter 'quit' to quit the game. The jumble word is: pmiarnogrmg User's first guess: prominggam Incorrect guess. Please try again! The first letter of the word is: p User's second guess: programming Congratulations! You have successfully unscrambled the word!
import java.util.Random; public class JumbleWordGame { // Function to jumble the word public static String jumbleWord(String word) { char[] jumbledWord = word.toCharArray(); Random rand = new Random(); for (int i = 0; i < jumbledWord.length - 1; i++) { int j = i + rand.nextInt(jumbledWord.length - i); char temp = jumbledWord[i]; jumbledWord[i] = jumbledWord[j]; jumbledWord[j] = temp; } return new String(jumbledWord); } public static void main(String[] args) { Random rand = new Random(); String originalWord = "programming"; String jumbledWord = jumbleWord(originalWord); // Simulate user actions String firstUserGuess = "prominggam"; String userHintRequest = "hint"; String secondUserGuess = "programming"; System.out.println("Welcome to the Jumble Word Game!"); System.out.println("Unscramble the letters to form a word."); System.out.println("Enter 'hint' for a hint."); System.out.println("Enter 'quit' to quit the game."); System.out.println("The jumble word is: " + jumbledWord); // Simulate the first user guess System.out.println("User's first guess: " + firstUserGuess); if (firstUserGuess.equals(originalWord)) { System.out.println("Congratulations! You have successfully unscrambled the word!"); } else { System.out.println("Incorrect guess. Please try again!"); } // Simulate user asking for a hint if (userHintRequest.equals("hint")) { System.out.println("The first letter of the word is: " + originalWord.charAt(0)); } // Simulate the second user guess System.out.println("User's second guess: " + secondUserGuess); if (secondUserGuess.equals(originalWord)) { System.out.println("Congratulations! You have successfully unscrambled the word!"); } else { System.out.println("Incorrect guess. Please try again!"); } } }
Output
Welcome to the Jumble Word Game! Unscramble the letters to form a word. Enter 'hint' for a hint. Enter 'quit' to quit the game. The jumble word is: pmiarnogrmg User's first guess: prominggam Incorrect guess. Please try again! The first letter of the word is: p User's second guess: programming Congratulations! You have successfully unscrambled the word!
import random # Function to jumble the word def jumble_word(word): jumbled_word = list(word) random.shuffle(jumbled_word) return ''.join(jumbled_word) def main(): random.seed() original_word = "programming" jumbled_word = jumble_word(original_word) # Simulate user actions first_user_guess = "prominggam" user_hint_request = "hint" second_user_guess = "programming" print("Welcome to the Jumble Word Game!") print("Unscramble the letters to form a word.") print("Enter 'hint' for a hint.") print("Enter 'quit' to quit the game.") print(f"The jumble word is: {jumbled_word}") # Simulate the first user guess print(f"User's first guess: {first_user_guess}") if first_user_guess == original_word: print("Congratulations! You have successfully unscrambled the word!") else: print("Incorrect guess. Please try again!") # Simulate user asking for a hint if user_hint_request == "hint": print(f"The first letter of the word is: {original_word[0]}") # Simulate the second user guess print(f"User's second guess: {second_user_guess}") if second_user_guess == original_word: print("Congratulations! You have successfully unscrambled the word!") else: print("Incorrect guess. Please try again!") if __name__ == "__main__": main()
Output
Welcome to the Jumble Word Game! Unscramble the letters to form a word. Enter 'hint' for a hint. Enter 'quit' to quit the game. The jumble word is: pmiarnogrmg User's first guess: prominggam Incorrect guess. Please try again! The first letter of the word is: p User's second guess: programming Congratulations! You have successfully unscrambled the word!
Here's a sample test case for the Custom Jumble Word Game implemented above:
Suppose the original word is "programming". The program will generate a jumbled version of the word, for instance: "mgimnaprorg". The user's task is to unscramble the jumbled word and find the original word.
In this test case −
The program displays the jumbled word: mgimnaprorg.
The user enters their first guess: "prominggam" (Incorrect guess).
The user asks for a hint: The program reveals that the first letter of the word is "p".
The user enters another guess: "programming" (Correct guess).
The program congratulates the user for successfully unscrambling the word and exits.
This sample test case demonstrates how the Custom Jumble Word Game works and how the user can interact with the game by making guesses, asking for hints, or quitting the game.
Conclusion
In this article, we explored the exciting world of word puzzles by creating a custom Jumble Word Game in C++. We discussed the game's objective, C++ implementation, and provided a test case example to illustrate the game's functionality. By implementing this game, you not only enhance your programming skills but also engage in a fun and educational activity. You can further improve this game by adding more features like levels, categories, or time constraints to make it more challenging and enjoyable for players. Happy coding and gaming!