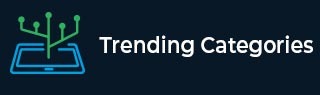
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Creating an Airbnb Rheostat Slider in React JS
In this article, we will see how to create an Airbnb rheostat. Rheostat is a www, mobile, and accessible slider component built with React. Rheostat is a scrollbar where you can slide the pointer to select some values or decide a range of values.
Example
First create a React project −
npx create-react-app tutorialpurpose
Go to the project directory −
cd tutorialpurpose
Download and install the rheostat package −
npm install rheostat
We can use this package to include premade rheostats with default functions inside a React project.
Insert the following lines of code in App.js −
import React from "react"; import Rheostat from "rheostat"; import "rheostat/initialize"; import "./App.css"; export default function App() { const [min, setMin] = React.useState(""); const [max, setMax] = React.useState(""); return ( <> <Rheostat min={1} max={100} values={[1, 100]} onValuesUpdated={(e) => { setMin(e.values[0]); setMax(e.values[1]); }} /> <div> <p>Min value:{min}</p> <p>Max value:{max}</p> </div> </> ); }
Here, the arguments, min and max, have the minimum and maximum values of the rheostat, respectively.
This will give you slider, but it actually does not look good, and we need to add some CSS to it. So we will use the default CSS that is given in its documentation.
Add the following lines of code in App.js −
.DefaultProgressBar__vertical { width: 24px; height: 100%; } .DefaultProgressBar_progressBar { background-color: #abc4e8; position: absolute } .DefaultProgressBar_progressBar__vertical { height: 100%; width: 24px } .DefaultProgressBar_background__vertical { height: 100%; top: 0px; width: 15px } .DefaultProgressBar_background__horizontal { height: 13px; top: 0px } .DefaultHandle_handle { width: 24px; height: 24px; border-width: 1px; border-style: solid; border-color: #d8d8d8; background-color: #fcfcfc; border-radius: 20%; outline: none; z-index: 2; box-shadow: 0 2px 2px #181616 } .DefaultHandle_handle:focus { box-shadow: #abc4e8 0 0 1px 1px } .DefaultHandle_handle:after { content: ""; display: block; position: absolute; background-color: #1f2124 } .DefaultHandle_handle:before { content: ""; display: block; position: absolute; background-color: #1f2124 } .DefaultHandle_handle__horizontal { margin-left: -12px; top: -5px } .DefaultHandle_handle__horizontal:before { top: 7px; height: 10px; width: 1px; left: 10px } .DefaultHandle_handle__horizontal:after { top: 7px; height: 10px; width: 1px; left: 13px } .DefaultHandle_handle__disabled { border-color: #dbdbdb } .DefaultBackground { background-color: #353434; height: 15px; width: 100%; border: 1px solid #d8d8d8; position: relative } .DefaultBackground_background__horizontal { height: 15px; top: -2px; left: -2px; bottom: 4px; width: 100% } .DefaultBackground_background__vertical { width: 15px; top: 0px; height: 100% } .rheostat { position: relative; overflow: visible; margin-top: 100px; } @media (min-width: 1128px) { .autoAdjustVerticalPosition { top: 12px } } .rheostat__vertical { height: 100% } .handleContainer { height: 15px; top: -2px; left: -2px; bottom: 4px; width: 100%; position: absolute } .rheostat_background { background-color: #fcfcfc; border: 1px solid #d8d8d8; position: relative } .rheostat_background__horizontal { height: 15px; top: -2px; left: -2px; bottom: 4px; width: 100% } .rheostat_background__vertical { width: 15px; top: 0px; height: 100% }
This is the whole CSS which will define the styles of all other components of the rheostat.
From the Chrome console, check the element names, and try to change some CSS values and check the corresponding output.
Output
On execution, it will produce the following output −