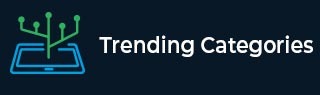
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Creating 3D Text using React-three-fiber
In this article, we will see how to create a 3D text using react-threefiber. We will first download the font of JSON and then we will add it in our Text Geometry object. We will add orbit control to it which will allow moving the text on the screen and view the 3D text properly. So, let's get started.
Example
First, download important libraries −
npm i --save @react-three/fiber three
This library react-three/fiber will be used to add webGL renderer to the website and to connect threejs and React.
Now install a typeface font JSON and put it inside “src” folder. You can download a font from google fonts and go to https://gero3.github.io/facetype.js/ for converting it into JSON.
Add the following lines of code in App.js −
import React, { useEffect, useRef } from "react"; import { Canvas, useThree ,useLoader } from "@reactthree/fiber"; import { OrbitControls } from "three/examples/jsm/controls/OrbitControls"; import * as THREE from "three"; import Roboto from "./Roboto_Regular.json" import "./App.css"; const CameraController = () => { const { camera, gl } = useThree(); useEffect( () => { const controls = new OrbitControls(camera, gl.domElement); controls.minDistance = 3; controls.maxDistance = 20; return () => { controls.dispose(); }; }, [camera, gl] ); return null; }; function Text3d(){ const font = new THREE.FontLoader().parse(Roboto); const textOptions = { font, size: 5, height: 1 }; return ( <mesh> <textGeometry attach='geometry' args={['three.js', text Options]} /> <meshStandardMaterial attach='material' color="hotpink" /> </mesh> ) } export default function App(){ return ( <Canvas> <CameraController/> <ambientLight /> <Text3d/> </Canvas> ); };
Explanation
We first loaded the JSON font with “Roboto” variable and then inside our function, we parsed it as a font and stored it in “font” const.
Then we set its styles and other aspects in “textOptions”. Next we simply created textGeometry and passed args, and material object is used to apply styles.
Output
It will produce the following output −