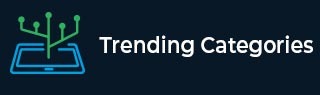
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Create Array of Zeros in MATLAB
In MATLAB, the variables are typically stored in the form of a matrix or array. Sometimes, we require a matrix or array of zeros to perform a specific operation. Therefore, MATLAB allows us to create a matrix or array of zeros. For this task, we can use MATLAB's built-in functions or manual methods.
In this article, we will learn different methods to create a matrix or array of zero with the help of example programs in MATLAB programming.
Array of Zeros using `zeros()` Function in MATLAB
MATLAB provides a built-in function named `zeros()` to create a matrix or array of zeros with a specific number of rows and columns.
Creating an array of zeros with specified number of rows and columns
We can use the `zeros()` function to create an array of n × m order by using the following syntax.
Syntax
A = zeros(n, m);
Here, `A` is a variable defined to store the array of zeros, `n` is a number representing number of rows in the array, and `m` is the number representing number of columns in the array.
Example
% MATLAB program to demonstrate the creation of an n x m array of zeros using `zeros()` function % Creating a 3 x 4 array of zeros A = zeros (3, 4); % Display the array of zeros disp('The array of zeros: '); disp(A);
Output
The array of zeros: 0 0 0 0 0 0 0 0 0 0 0 0
Creating an array of zeros of with same number of rows and columns
The following syntax of the `zeros()` function is used to create an n × n array of zeros.
Syntax
A = zeros(n);
Where, `n` is the order of the array.
Example
% MATLAB program to demonstrate the creation of an n x n array of zeros % Creating a 3 x 3 array of zeros A = zeros (3); % Display the array of zeros disp('The array of zeros: '); disp(A);
Output
The array of zeros: 0 0 0 0 0 0 0 0 0
Creating a scalar zero
We can create a scalar zero just by calling the `zeros` function without arguments. The following MATLAB program demonstrates the implementation of creating a scalar zero.
Example
% MATLAB program to demonstrate the creation of a scalar zero % Creating a scalar zero A = zeros; % Display the scalar zero disp('The scalar zero is: '); disp(A);
Output
The scalar zero is: 0
Creating a 3-d array of zeros
We can also use the `zeros()` function with three arguments to create a 3-d array of zeros. For this, the zeros() function will take the following syntax.
Syntax
A = zeros (a, b, c);
This function will create an a x b x c array of zeros.
Example
% MATLAB program to demonstrate the creation of a 3-d array of zeros % Creating a 3 x 4 x 5 array of zeros A = zeros (3, 4, 5); % Display the array of zeros disp('The array of zeros: '); disp(A);
Output
The array of zeros: (:,:,1) = 0 0 0 0 0 0 0 0 0 0 0 0 (:,:,2) = 0 0 0 0 0 0 0 0 0 0 0 0 (:,:,3) = 0 0 0 0 0 0 0 0 0 0 0 0 (:,:,4) = 0 0 0 0 0 0 0 0 0 0 0 0 (:,:,5) = 0 0 0 0 0 0 0 0 0 0 0 0
Create Array of Zeros Manually in MATLAB
We can specify the codes in MATLAB programming to create different kinds of arrays of zeros. The following sections illustrates the creation of arrays of zeros manually.
Create an array of zeros of size 1 x n or an array of n zeros
We can create a row vector of size 1 x n or an array of n zeros in the MATLAB programming.
The following MATLAB program demonstrate the creation of an array of 6 (n = 6) zeros.
Example
% MATLAB program to demonstrate the creation of an array of n zeros % Creating an array of 6 (n = 6) zeros A = [0 0 0 0 0 0]; % Display the array of zeros disp('The array of zeros is: '); disp(A);
Output
The array of zeros is: 0 0 0 0 0 0
Create an array of zeros of size n x 1 or a column vector of zeros
We can create a column vector of size n x 1 or an array of zeros of size n x 1 in the MATLAB programming.
The following MATLAB program demonstrate the creation of an array of zeros of size 6 x 1.
Example
% MATLAB program to demonstrate the creation of an n x 1 array of zeros % Creating an array of zeros of size 6 x 1 A = [0; 0; 0; 0; 0; 0]; % Display the array of zeros disp('The array of zeros is: '); disp(A);
Output
The array of zeros is: 0 0 0 0 0 0
Create an array of zeros of size n x m
We can create an array of zeros of size n x m in the MATLAB programming. Where, `n` is the number of rows and `m` is the number of columns in the array.
The following MATLAB program demonstrate the creation of an array of zeros of size n x m.
Example
% MATLAB program to demonstrate the creation of an n x m array of zeros % Creating an array of zeros of size 3 x 5 A = [0 0 0 0 0; 0 0 0 0 0; 0 0 0 0 0]; % Display the array of zeros disp('The array of zeros is: '); disp(A);
Output
The array of zeros is: 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0
Conclusion
In conclusion, we can create array of zeros in MATALB either manually or by using built-in function “zeros()”. The above MATLAB programs demonstrate both methods of creating array of zeros.