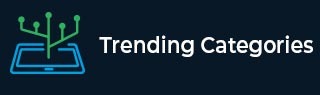
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Create an array with ones at and below the given diagonal and zeros elsewhere with a different output type in Numpy
To create an array with ones at and below the given diagonal and zeros elsewhere, use the numpy.tri() method in Python Numpy
- The 1st parameter is the number of rows in the array
- The 2nd parameter is the number of columns in the array
- The "type" parameter is used to set the type of the returned array
The tri() function returns an array with its lower triangle filled with ones and zero elsewhere; in other words T[i,j] == 1 for j <= i + k, 0 otherwise.
Steps
At first, import the required library −
import numpy as np
Now, create an array with ones at and below the given diagonal and zeros elsewhere, using the numpy.tri() method −
arr = np.tri(3, 5, dtype = int)
Displaying our array −
print("Array...
",arr)
Get the datatype −
print("
Array datatype...
",arr.dtype)
Get the dimensions of the Array −
print("
Array Dimensions...
",arr.ndim)
Get the shape of the Array −
print("
Our Array Shape...
",arr.shape)
Get the number of elements of the Array −
print("
Elements in the Array...
",arr.size)
Example
import numpy as np # To create an array with ones at and below the given diagonal and zeros elsewhere, use the numpy.tri() method in Python Numpy # The 1st parameter is the number of rows in the array # The 2nd parameter is the number of columns in the array # The "type" parameter is used to set the type of the returned array arr = np.tri(3, 5, dtype = int) # Displaying our array print("Array...
",arr) # Get the datatype print("
Array datatype...
",arr.dtype) # Get the dimensions of the Array print("
Array Dimensions...
",arr.ndim) # Get the shape of the Array print("
Our Array Shape...
",arr.shape) # Get the number of elements of the Array print("
Elements in the Array...
",arr.size)
Output
Array... [[1 0 0 0 0] [1 1 0 0 0] [1 1 1 0 0]] Array datatype... int64 Array Dimensions... 2 Our Array Shape... (3, 5) Elements in the Array... 15
Advertisements