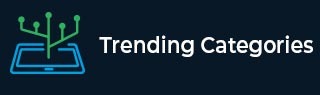
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Create a string of specific length in C++
In C++, the string is a collection of various alphanumeric and special characters. We can create a string using the ‘string’ data type in C++.
Problem Statement
We have given a length of the string and one single character, and we require to generate a string of a given length containing the single character.
In C++, we can define the string of a particular length by hard coding the values, but when we require to generate a string of different lengths and use the given character, we require to use the below approaches.
Sample Examples
Here are the sample examples for the above problem statement.
Input
len = 5, character = ‘p’
Output
‘PPPPP’
Explanation
It generates a string of length 5 using the ‘P’ character.
Input
len = 10, character = ‘L’
Output
‘LLLLLLLLLL’
Explanation
It generates a string of length 10 using the ‘L’ character.
Use the below approaches to solve the above problem.
Using the String() Constructor
In C++, the string() constructor allows us to create a string of a particular length using a particular character. It takes a length as the first parameter and a character as the second parameter.
Syntax
Users can follow the syntax below to use the string() constructor.
string str(Len, character);
Parameters
Len − It is the length of the string.
Character − It is a character to generate a string.
Example
In the example below, we have taken the ‘5’ as the length of the string and ‘a’ as the character of the string. After that, we have used the string() constructor and generated a string of length 5.
In the output, we can observe that string contains a total of 5 ‘a’ characters.
#include <bits/stdc++.h> using namespace std; int main(){ int Len; char character; Len = 5; character = 'a'; cout << "Entered string length is " << Len << endl; cout << "Entered character is " << character << endl; // use the string() constructor string str(Len, character); // print the string cout << "Resultant string is " << str; return 0; }
Output
Entered string length is 5 Entered character is a Resultant string is aaaaa
Time complexity − O(Len), as the constructor requires to make iterations to create a string.
Space complexity − O(Len), as it takes a space according to the length of the string.
Using the append() method
In C++, the append() method allows us to append the number of characters to the string. For example, we can append different characters or a single character multiple times to the string.
Syntax
Users can follow the syntax below to use the append() method to generate a string of length N.
str.append(Len, character);
The append() method takes the total number of characters as a first parameter and the character as a second parameter.
Example
In this example, we have taken 20 as a string length and ‘j’ as a string character. After that, we have used the append() method to append the ‘j’ character 20 times to the ‘str’ string.
In the output, we can observe the string containing a total of 20 ‘j’ characters.
#include <iostream> using namespace std; int main(){ int Len; char character; string str; Len = 20; character = 'j'; cout << "Entered string length is " << Len << endl; cout << "Entered character is " << character << endl; // using the append function to add a character str.append(Len, character); // print the string cout << "Resultant string is " << str; return 0; }
Output
Entered string length is 20 Entered character is j Resultant string is jjjjjjjjjjjjjjjjjjjj
Time complexity − O(Len).
Space complexity − O(Len).
Using the for loop
In C++, we can use the for loop to make iterations. Here, we can use the ‘+’ operator to append the single character to the string in each iteration of for loop. After completing the N iterations of for loop, we can generate a string of length N.
Syntax
Users can follow the syntax below to use the for loop to generate a string of length N.
for (int i = 0; i < Len; i++){ str = str + character; }
In the above syntax, we append the character to the string.
Example
In the example below, we have taken 30 as a string length and ‘8’ as a character. After that, we make a total ‘Len’ number of iterations of for loop, and in each iteration, we append ‘8’ to the ‘str’.
In the output, we can see the string containing the 30 ‘8’ characters.
#include <iostream> using namespace std; int main(){ int Len; char character; string str; Len = 30; character = '8'; cout << "Entered string length is " << Len << endl; cout << "Entered character is " << character << endl; // using the append function to add a character // using the for loop for (int i = 0; i < Len; i++) { str = str + character; } // print the string cout << "Resultant string is " << str; return 0; }
Output
Entered string length is 30 Entered character is 8 Resultant string is 888888888888888888888888888888
Time complexity − O(Len), as we make a Len number of iterations using the for loop.
Space complexity − O(Len).
Conclusion
In this tutorial, we learned three different approaches to generating a length N containing a single character. The first approach uses the string() constructor, the second approach uses the append() method, and the third one uses the for loop.
Here, all three approaches have the same time and space complexity, but using the string constructor makes the code more readable.