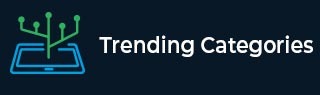
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Create a sample of data frame column by excluding NAs in R
To create a random sample by excluding missing values of a data frame column, we can use sample function and the negation of is.na with the column of the data frame.
For Example, if we have a data frame called df that contains a column X which has some NAs then we can create a random sample of size 100 of X values by using the following command −
sample(df$X[!is.na(df$X)],100,replace=TRUE).
Example 1
Following is the code snippet to create dataframe −
x<-rep(c(NA,2,5,10,15),times=4) df1<-data.frame(x) df1
The following dataframe is created −
By the x 1 NA 2 2 3 5 4 10 5 15 6 NA 7 2 8 5 9 10 10 15 11 NA 12 2 13 5 14 10 15 15 16 NA 17 2 18 5 19 10 20 15
To create a random sample of x of size 100 by excluding NAs on the above created data frame, add the above code to the following snippet −
x<-rep(c(NA,2,5,10,15),times=4) df1<-data.frame(x) sample(df1$x[!is.na(df1$x)],100,replace=TRUE)
Output
If you execute all the above given snippets as a single program, it generates the following Output −
[1] 10 10 5 10 5 2 2 2 15 2 2 5 10 10 2 15 10 10 2 5 2 2 10 2 10 [26] 15 2 10 10 2 10 5 2 15 15 10 5 2 5 2 15 5 10 10 10 10 5 15 2 10 [51] 10 15 5 10 15 10 2 10 15 15 15 10 15 15 2 5 5 15 2 15 15 5 2 2 5 [76] 5 2 10 2 10 2 15 10 5 15 2 10 5 15 15 15 10 2 10 5 15 5 5 15 2
Example 2
Following snippet creates a sample data frame −
y<-rep(c(NA,rnorm(1),rnorm(1),rnorm(1)),times=5) df2<-data.frame(y) df2
The following dataframe is created −
y 1 NA 2 -1.2548971 3 1.1956757 4 0.6556753 5 NA 6 -1.2548971 7 1.1956757 8 0.6556753 9 NA 10 -1.2548971 11 1.1956757 12 0.6556753 13 NA 14 -1.2548971 15 1.1956757 16 0.6556753 17 NA 18 -1.2548971 19 1.1956757 20 0.6556753
To create a random sample of y of size 100 by excluding NAs on the above created data frame, add the following code to the above snippet −
y<-rep(c(NA,rnorm(1),rnorm(1),rnorm(1)),times=5) df2<-data.frame(y) sample(df2$y[!is.na(df2$y)],50,replace=TRUE)
Output
If you execute all the above given snippets as a single program, it generates the following Output −
[1] 0.6556753 -1.2548971 0.6556753 1.1956757 0.6556753 0.6556753 [7] -1.2548971 0.6556753 0.6556753 0.6556753 -1.2548971 1.1956757 [13] 0.6556753 -1.2548971 -1.2548971 -1.2548971 0.6556753 1.1956757 [19] -1.2548971 -1.2548971 0.6556753 -1.2548971 1.1956757 1.1956757 [25] 0.6556753 0.6556753 1.1956757 1.1956757 -1.2548971 0.6556753 [31] 0.6556753 1.1956757 0.6556753 1.1956757 0.6556753 0.6556753 [37] 0.6556753 -1.2548971 1.1956757 0.6556753 0.6556753 -1.2548971 [43] -1.2548971 0.6556753 1.1956757 0.6556753 -1.2548971 1.1956757 [49] -1.2548971 -1.2548971
Example 3
Following snippet creates a sample data frame −
z<-rep(c(NA,rpois(1,5),rpois(1,2),rpois(1,10),rpois(1,3)),times=4) df3<-data.frame(z) df3
The following dataframe is created −
z 1 NA 2 7 3 2 4 10 5 1 6 NA 7 7 8 2 9 10 10 1 11 NA 12 7 13 2 14 10 15 1 16 NA 17 7 18 2 19 10 20 1
To create a random sample of z of size 100 by excluding NAs on the above created data frame, add the following code to the above snippet −
z<-rep(c(NA,rpois(1,5),rpois(1,2),rpois(1,10),rpois(1,3)),times=4) df3<-data.frame(z) sample(df3$z[!is.na(df3$z)],200,replace=TRUE)
Output
If you execute all the above given snippets as a single program, it generates the following Output −
[1] 10 2 2 2 7 2 1 2 10 2 10 2 1 1 7 1 10 2 10 1 2 10 7 1 7 [26] 1 2 10 2 2 10 10 2 7 10 7 7 7 10 2 1 2 2 10 2 2 10 10 7 7 [51] 1 7 1 10 2 10 7 2 7 2 10 2 1 7 7 7 2 2 10 10 10 10 7 7 2 [76] 2 2 1 1 7 7 7 2 1 7 1 2 10 10 2 10 10 10 7 2 10 10 2 10 7 [101] 7 10 7 2 10 2 10 10 7 10 2 2 2 1 1 1 7 10 7 10 7 7 2 2 7 [126] 10 2 2 2 2 1 10 1 2 7 10 10 1 10 10 7 7 2 2 7 2 2 1 2 10 [151] 7 2 7 10 10 1 10 7 2 7 2 7 1 10 7 2 2 2 1 10 10 2 10 1 1 [176] 7 10 1 10 1 1 2 2 1 2 10 1 10 7 7 2 7 10 10 1 10 1 1 1 7