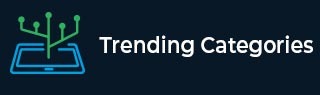
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Create a Pandas Dataframe from a dict of equal length lists in Python
The Dataframe in pandas can be created using various options. One of the option is to take a dictionary and convert it to a Dataframe. In this article we will see how to take three lists of equal length and convert them to a pandas dataframe using a python dictionary.
Uisng Lists and Dictionary
In this approach we have the lists declared individually. Then each of them is used as a value for the appropriate key inside a dictionary definition. Finally the pandas method called pd.Dataframe is applied to the dictionary.
Example
import pandas as pd # Lists for Exam schedule Days = ['Mon', 'Tue', 'Wed','Thu', 'Fri'] Sub = ['Chemisry','Physics','Maths','English','Biology'] Time = ['2 PM', '10 AM', '11 AM','1 PM', '3 PM'] # Dictionary for Exam Schedule Exam_Schedule = {'Exam Day': Days, 'Exam Subject': Sub, 'Exam Time': Time} # Dictionary to DataFrame Exam_Schedule_df = pd.DataFrame(Exam_Schedule) print(Exam_Schedule_df)
Output
Running the above code gives us the following result −
Exam Day Exam Subject Exam Time 0 Mon Chemisry 2 PM 1 Tue Physics 10 AM 2 Wed Maths 11 AM 3 Thu English 1 PM 4 Fri Biology 3 PM
Using Lists inside a Dictionary
In this approach we take the lists directly as the values inside the dictionary instead of declaring them individually. Then the dictionary is converted to the pandas dataframe in a similar manner as above.
Example
import pandas as pd # Dictionary for Exam Schedule Exam_Schedule = { 'Exam Day': ['Mon', 'Tue', 'Wed','Thu', 'Fri'], 'Exam Subject': ['Chemisry','Physics','Maths','English','Biology'], 'Exam Time': ['2 PM', '10 AM', '11 AM','1 PM', '3 PM'] } # Dictionary to DataFrame Exam_Schedule_df = pd.DataFrame(Exam_Schedule) print(Exam_Schedule_df)
Output
Running the above code gives us the following result −
Exam Day Exam Subject Exam Time 0 Mon Chemisry 2 PM 1 Tue Physics 10 AM 2 Wed Maths 11 AM 3 Thu English 1 PM 4 Fri Biology 3 PM