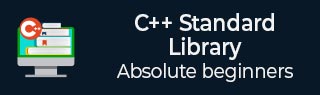
- The C Standard Library
- The C Standard Library
- The C++ Standard Library
- C++ Library - Home
- C++ Library - <fstream>
- C++ Library - <iomanip>
- C++ Library - <ios>
- C++ Library - <iosfwd>
- C++ Library - <iostream>
- C++ Library - <istream>
- C++ Library - <ostream>
- C++ Library - <sstream>
- C++ Library - <streambuf>
- C++ Library - <atomic>
- C++ Library - <complex>
- C++ Library - <exception>
- C++ Library - <functional>
- C++ Library - <limits>
- C++ Library - <locale>
- C++ Library - <memory>
- C++ Library - <new>
- C++ Library - <numeric>
- C++ Library - <regex>
- C++ Library - <stdexcept>
- C++ Library - <string>
- C++ Library - <thread>
- C++ Library - <tuple>
- C++ Library - <typeinfo>
- C++ Library - <utility>
- C++ Library - <valarray>
- The C++ STL Library
- C++ Library - <array>
- C++ Library - <bitset>
- C++ Library - <deque>
- C++ Library - <forward_list>
- C++ Library - <list>
- C++ Library - <map>
- C++ Library - <multimap>
- C++ Library - <queue>
- C++ Library - <priority_queue>
- C++ Library - <set>
- C++ Library - <stack>
- C++ Library - <unordered_map>
- C++ Library - <unordered_set>
- C++ Library - <vector>
- C++ Library - <algorithm>
- C++ Library - <iterator>
- C++ Programming Resources
- C++ Programming Tutorial
- C++ Useful Resources
- C++ Discussion
C++ Vector Library - insert() Function
Description
The C++ function std::vector::insert() extends vector by inserting new elements in the container. Reallocation happens if there is need of more space
This function increases container size.
Declaration
Following is the declaration for std::vector::insert() function form std::vector header.
C++98
template <class InputIterator> void insert (iterator position, InputIterator first, InputIterator last);
C++11
template <class InputIterator> iterator insert (const_iterator position, InputIterator first, InputIterator last);
Parameters
position − Index in the vector where new element to be inserted.
first − Input iterator to the initial position in range.
last − Input iterator to the final position in range.
Return value
Returns an iterator which points to the newly inserted element.
Time complexity
Linear i.e. O(n)
Example
The following example shows the usage of std::vector::insert() function.
#include <iostream> #include <vector> using namespace std; int main(void) { vector<int> v1 = {2, 3, 4, 5}; vector<int> v2 = {1}; v2.insert(v2.begin() + 1, v1.begin(), v1.begin() + 3); for (auto it = v2.begin(); it != v2.end(); ++it) cout << *it << endl; return 0; }
Let us compile and run the above program, this will produce the following result −
1 2 3 4
vector.htm
Advertisements