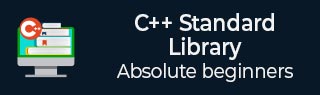
- The C Standard Library
- The C Standard Library
- The C++ Standard Library
- C++ Library - Home
- C++ Library - <fstream>
- C++ Library - <iomanip>
- C++ Library - <ios>
- C++ Library - <iosfwd>
- C++ Library - <iostream>
- C++ Library - <istream>
- C++ Library - <ostream>
- C++ Library - <sstream>
- C++ Library - <streambuf>
- C++ Library - <atomic>
- C++ Library - <complex>
- C++ Library - <exception>
- C++ Library - <functional>
- C++ Library - <limits>
- C++ Library - <locale>
- C++ Library - <memory>
- C++ Library - <new>
- C++ Library - <numeric>
- C++ Library - <regex>
- C++ Library - <stdexcept>
- C++ Library - <string>
- C++ Library - <thread>
- C++ Library - <tuple>
- C++ Library - <typeinfo>
- C++ Library - <utility>
- C++ Library - <valarray>
- The C++ STL Library
- C++ Library - <array>
- C++ Library - <bitset>
- C++ Library - <deque>
- C++ Library - <forward_list>
- C++ Library - <list>
- C++ Library - <map>
- C++ Library - <multimap>
- C++ Library - <queue>
- C++ Library - <priority_queue>
- C++ Library - <set>
- C++ Library - <stack>
- C++ Library - <unordered_map>
- C++ Library - <unordered_set>
- C++ Library - <vector>
- C++ Library - <algorithm>
- C++ Library - <iterator>
- C++ Programming Resources
- C++ Programming Tutorial
- C++ Useful Resources
- C++ Discussion
C++ Vector Library - vector() Function
Description
The C++ copy constructor std::vector::vector() constructs a container with copy of each elements present in existing container x.
Declaration
Following is the declaration for copy constructor std::vector::vector() form std::vector header.
C++98
vector (const vector& x);
C++11
vector (const vector& x, const allocator_type& alloc);
Parameters
x − Another vector container of same type.
Return value
Constructor never returns value.
Exceptions
This member function never throws exception.
Time complexity
Linear i.e. O(n)
Example
The following example shows the usage of copy constructor std::vector::vector().
#include <iostream> #include <vector> using namespace std; int main(void) { vector<int> v1(5); /* assign value to vector v1 */ for (int i = 0; i < v1.size(); ++i) v1[i] = i + 1; /* create a copy constructor v2 from v1 */ vector<int> v2(v1); for (int i = 0; i < v2.size(); ++i) cout << v2[i] << endl; return 0; }
Let us compile and run the above program, this will produce the following result −
1 2 3 4 5
vector.htm
Advertisements