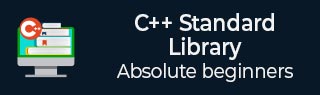
- The C Standard Library
- The C Standard Library
- The C++ Standard Library
- C++ Library - Home
- C++ Library - <fstream>
- C++ Library - <iomanip>
- C++ Library - <ios>
- C++ Library - <iosfwd>
- C++ Library - <iostream>
- C++ Library - <istream>
- C++ Library - <ostream>
- C++ Library - <sstream>
- C++ Library - <streambuf>
- C++ Library - <atomic>
- C++ Library - <complex>
- C++ Library - <exception>
- C++ Library - <functional>
- C++ Library - <limits>
- C++ Library - <locale>
- C++ Library - <memory>
- C++ Library - <new>
- C++ Library - <numeric>
- C++ Library - <regex>
- C++ Library - <stdexcept>
- C++ Library - <string>
- C++ Library - <thread>
- C++ Library - <tuple>
- C++ Library - <typeinfo>
- C++ Library - <utility>
- C++ Library - <valarray>
- The C++ STL Library
- C++ Library - <array>
- C++ Library - <bitset>
- C++ Library - <deque>
- C++ Library - <forward_list>
- C++ Library - <list>
- C++ Library - <map>
- C++ Library - <multimap>
- C++ Library - <queue>
- C++ Library - <priority_queue>
- C++ Library - <set>
- C++ Library - <stack>
- C++ Library - <unordered_map>
- C++ Library - <unordered_set>
- C++ Library - <vector>
- C++ Library - <algorithm>
- C++ Library - <iterator>
- C++ Programming Resources
- C++ Programming Tutorial
- C++ Useful Resources
- C++ Discussion
C++ Unordered_multimap::clear() Function
The C++ std::unordered_multimap::clear() function is used to destroys or drop the unordered_multimap by removing all elements and sets the size of unordered_multimap to zero.
After removing all the elements from the unordered_multimap, we can add the new element to the same unordered_multimap using the insert() and emplace() function.
Syntax
Following is the syntax of std::unordered_multimap::clear() function.
void clear() noexcept;
Parameters
This function does not accepts any parameters.
Return value
This function does not returns anything because the size of unordered_multimap after using the clear() function is zero.
Example 1
In the following example, let's see the usage of clear() function.
#include <iostream> #include <unordered_map> using namespace std; int main(void) { unordered_multimap<char, int> umm = { {'a', 1}, {'b', 2}, {'c', 3}, {'b', 2}, {'c', 3}, {'d', 4}, }; cout << "Initial size of unordered multimap = " << umm.size() << endl; umm.clear(); cout << "Size of unordered multimap after use of clear() function = " << umm.size() << endl; return 0; }
Output
Let us compile and run the above program, this will produce the following result −
Initial size of unordered multimap = 5 Size of unordered multimap after clear operaion = 0
Example 2
consider the following example, where we are going to add the elements into the map after performing the clear() function.
#include <iostream> #include <string> #include <unordered_map> using namespace std; int main () { unordered_multimap<string,string> ummap = { {"Aman","Kumar"}, {"Vivek","Verma"}, {"Jhon","Satya"} }; cout << "ummap contains:"; for (auto& x: ummap)cout << " " << x.first << "=" << x.second; cout << endl; //removing all the element from the map. ummap.clear(); //adding two element from the map ummap.insert({"tutorialspoint", "Noida"}); ummap.insert({"tutorialspoint","Hyderabad"}); cout << "ummap contains:"; for (auto& x: ummap) cout << " " << x.first << "=" << x.second; cout << endl; return 0; }
Output
If we run the above code it will generate the following output −
ummap contains: Vivek=Verma Jhon=Satya Aman=Kumar ummap contains: tutorialspoint=Hyderabad tutorialspoint=Noida
Example 3
Let's look at the following example, where we are going to display the elements of unordered_multimap later, applying the clear() function and displaying the size of the unordered_multimap.
#include <iostream> #include <string> #include <unordered_map> using namespace std; int main () { unordered_multimap<int, string> uMmap; uMmap.insert({1, "tutorialspoint"}); uMmap.insert({1, "tutorialspoint"}); uMmap.insert({2, "Hyderabad India"}); uMmap.insert({3, "Tutorix"}); uMmap.insert({4, "Noida India"}); cout<<"Before use of clear() functon"; for (auto& x: uMmap) cout << "\n" << x.first << "=" << x.second; cout << endl; uMmap.clear(); cout<<"After use of clear() function \n"; cout <<uMmap.size(); return 0; }
Output
Following is the output of the above code −
Before use of clear() functon 4=Noida India 3=Tutorix 2=Hyderabad India 1=tutorialspoint 1=tutorialspoint After use of clear() function 0
Example 4
Following is the example, where we are going to use the to unordered_multimaps one stores the items, the other one stores the items obtained after filtering even keys from the first, later we apply the clear() function and display displays the size before and after.
#include <string> #include <iostream> #include <unordered_map> using namespace std; int main () { unordered_multimap<int, string> uMmap, umm; uMmap.insert({1, "tutorialspoint"}); uMmap.insert({1, "tutorialspoint"}); uMmap.insert({2, "Hyderabad India"}); uMmap.insert({3, "Tutorix"}); uMmap.insert({4, "Noida India"}); cout<<"Umm Before use of clear() functon"<<endl; for (auto& x: uMmap){ if(x.first % 2 != 0){ umm.insert({x.first, x.second}); } } for(auto& x: umm){ cout<<x.first<<" : "<<x.second<<endl; } cout<<"size of umm before use of clear function: "<<endl; cout <<umm.size()<<endl; cout<<"size of umm after use of clear() function \n"; // umm.clear(); umm.clear(); cout<<umm.size()<<endl; return 0; }
Output
Output of the above code is as follows −
Umm Before use of clear() functon 1 : tutorialspoint 1 : tutorialspoint 3 : Tutorix size of umm before use of clear function: 3 size of umm after use of clear() function 0