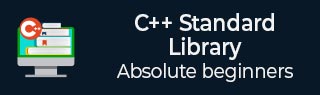
- The C Standard Library
- The C Standard Library
- The C++ Standard Library
- C++ Library - Home
- C++ Library - <fstream>
- C++ Library - <iomanip>
- C++ Library - <ios>
- C++ Library - <iosfwd>
- C++ Library - <iostream>
- C++ Library - <istream>
- C++ Library - <ostream>
- C++ Library - <sstream>
- C++ Library - <streambuf>
- C++ Library - <atomic>
- C++ Library - <complex>
- C++ Library - <exception>
- C++ Library - <functional>
- C++ Library - <limits>
- C++ Library - <locale>
- C++ Library - <memory>
- C++ Library - <new>
- C++ Library - <numeric>
- C++ Library - <regex>
- C++ Library - <stdexcept>
- C++ Library - <string>
- C++ Library - <thread>
- C++ Library - <tuple>
- C++ Library - <typeinfo>
- C++ Library - <utility>
- C++ Library - <valarray>
- The C++ STL Library
- C++ Library - <array>
- C++ Library - <bitset>
- C++ Library - <deque>
- C++ Library - <forward_list>
- C++ Library - <list>
- C++ Library - <map>
- C++ Library - <queue>
- C++ Library - <set>
- C++ Library - <stack>
- C++ Library - <unordered_map>
- C++ Library - <unordered_set>
- C++ Library - <vector>
- C++ Library - <algorithm>
- C++ Library - <iterator>
- C++ Programming Resources
- C++ Programming Tutorial
- C++ Useful Resources
- C++ Discussion
C++ Unordered_map::emplace() Function
The C++ function unordered_map::emplace() is used to insert new element into the container and increases the container size by one. The insertion will only takes place if the key doesn't exists in the container making it unique.
If the same key is emplaced multiple times, the map stores the first element only because the map is a container that does not store multiple keys of the same value.
Syntax
Following is the syntax of unordered_map::emplace() function
pair<iterator, bool> emplace( Args&&... args);
Parameters
args − It indicates the arguments to forward to the constructor of the element.
Return value
Returns a pair consisting of a bool to indicate whether insertion has happened or not and returns an iterator to the newly inserted element.
Example 1
Let's look at the basic usage of unordered_map::emplace() function.
#include <iostream> #include <unordered_map> using namespace std; int main(void) { unordered_map<char, int> um; um.emplace('a', 1); um.emplace('b', 2); um.emplace('c', 3); um.emplace('d', 4); um.emplace('e', 5); cout << "Unordered map contains following elements" << endl; for (auto it = um.begin(); it != um.end(); ++it) cout << it->first << " = " << it->second << endl; return 0; }
Output
Let us compile and run the above program, this will produce the following result −
Unordered map contains following elements e = 5 d = 4 c = 3 b = 2 a = 1
Example 2
In the following example, we created an unordered_map, then we are going to add two more keys and values using the emplace() function.
#include <iostream> #include <unordered_map> using namespace std; int main(void) { unordered_map<string, int> um = {{"Aman", 490},{"Vivek", 485},{"Akash", 500},{"Sonam", 450}}; cout << "Unordered map contains following elements before" << endl; for (auto it = um.begin(); it != um.end(); ++it) cout << it->first << " = " << it->second << endl; cout<<"after use of the emplace function \n"; um.emplace("Sarika", 440); um.emplace("Satya", 460); cout << "Unordered map contains following elements" << endl; for (auto it = um.begin(); it != um.end(); ++it) cout << it->first << " = " << it->second << endl; return 0; }
Output
If we run the above code it will generate the following output −
Unordered map contains following elements before Sonam = 450 Akash = 500 Vivek = 485 Aman = 490 after use of the emplace function Unordered map contains following elements Sarika = 440 Satya = 460 Sonam = 450 Akash = 500 Vivek = 485 Aman = 490
Example 3
Consider the following example, where we are trying to change the value of an present key using the emplace() function as follows:
#include <iostream> #include <unordered_map> using namespace std; int main(void) { unordered_map<string, int> um = {{"Aman", 490},{"Vivek", 485},{"Akash", 500},{"Sonam", 450}}; cout << "Unordered map contains following elements before usages of emplace" << endl; for (auto it = um.begin(); it != um.end(); ++it) cout << it->first << " = " << it->second << endl; um.emplace("Aman", 440); um.emplace("Sonam", 460); cout << "Unordered map contains same elements after the usages of emplace() function" << endl; for (auto it = um.begin(); it != um.end(); ++it) cout << it->first << " = " << it->second << endl; return 0; }
Output
Following is the output of the above code −
Unordered map contains following elements before Sonam = 450 Akash = 500 Vivek = 485 Aman = 490 Unordered map contains same elements after the usages of emplace() function Sonam = 450 Akash = 500 Vivek = 485 Aman = 490