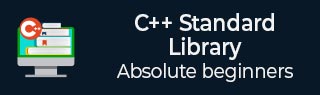
- The C Standard Library
- The C Standard Library
- The C++ Standard Library
- C++ Library - Home
- C++ Library - <fstream>
- C++ Library - <iomanip>
- C++ Library - <ios>
- C++ Library - <iosfwd>
- C++ Library - <iostream>
- C++ Library - <istream>
- C++ Library - <ostream>
- C++ Library - <sstream>
- C++ Library - <streambuf>
- C++ Library - <atomic>
- C++ Library - <complex>
- C++ Library - <exception>
- C++ Library - <functional>
- C++ Library - <limits>
- C++ Library - <locale>
- C++ Library - <memory>
- C++ Library - <new>
- C++ Library - <numeric>
- C++ Library - <regex>
- C++ Library - <stdexcept>
- C++ Library - <string>
- C++ Library - <thread>
- C++ Library - <tuple>
- C++ Library - <typeinfo>
- C++ Library - <utility>
- C++ Library - <valarray>
- The C++ STL Library
- C++ Library - <array>
- C++ Library - <bitset>
- C++ Library - <deque>
- C++ Library - <forward_list>
- C++ Library - <list>
- C++ Library - <map>
- C++ Library - <multimap>
- C++ Library - <queue>
- C++ Library - <priority_queue>
- C++ Library - <set>
- C++ Library - <stack>
- C++ Library - <unordered_map>
- C++ Library - <unordered_set>
- C++ Library - <vector>
- C++ Library - <algorithm>
- C++ Library - <iterator>
- C++ Programming Resources
- C++ Programming Tutorial
- C++ Useful Resources
- C++ Discussion
C++ Unordered_map::at() Function
The C++ unordered_map::at() function is used to retrieve the reference to the mapped value of the given item with key equivalent to key, or we can say that it returns the reference to the value with the element as key "k".
If the item does not exist, then an exception of type "out of range" is thrown. By using this function we can access the mapped value and modify them.
Syntax
Following is the syntax for std::unordered_map::at() function.
name_of_Unordered_map.at(k)
Parameters
k − Key value whose mapped value is accessed.
Return value
If object is constant qualified then method returns constant reference to mapped value otherwise returns non-constant reference.
Example 1
Let's consider the following example, where we are going to demonstrate the usage of std::unordered_map::at() function.
#include <iostream> #include <unordered_map> using namespace std; int main(void) { unordered_map<char, int> um = { {'a', 1}, {'b', 2}, {'c', 3}, {'d', 4}, {'e', 5} }; cout << "Value of key um['a'] = " << um.at('a') << endl; try { um.at('z'); } catch(const out_of_range &e) { cerr << "Exception at " << e.what() << endl; } return 0; }
Output
Let us compile and run the above program, this will produce the following result −
Value of key um['a'] = 1 Exception at _Map_base::at
Example 2
In the following example, we are going to return the key that doesn't exists in the map and observing the output.
#include <iostream> #include <unordered_map> using namespace std; int main(void) { unordered_map<char, int> um = { {'a', 1}, {'b', 2}, {'c', 3}, {'d', 4}, {'e', 5} }; cout << "Value of key um['z'] = " << um.at('z') << endl; return 0; }
Output
If we run the above code it will generate the following output −
terminate called after throwing an instance of 'std::out_of_range' what(): _Map_base::atAborted
Example 3
Consider the following example, where we are creating an unordered map that stores the marks of a student, and updating the marks using the at() function.
#include <iostream> #include <unordered_map> using namespace std; int main() { unordered_map<string,int> stu_marks = { {"Aman", 95}, {"Vivek", 90}, {"Sarika", 85}, {"Ammu", 100} }; stu_marks.at("Aman") = 100; stu_marks.at("Vivek") += 95; stu_marks.at("Sarika") += 92; for (auto& i: stu_marks) { cout<<i.first<<": "<<i.second<<endl; } return 0; }
Output
Following is the output of the above code −
Ammu: 100 Sarika: 177 Vivek: 185 Aman: 100
Example 4
Following is the example, where we are declaring an unordered map that has an integer-type key and a char-type value and observing the output.
#include <iostream> #include <unordered_map> using namespace std; int main() { unordered_map<int,char> ASCII = { {65, 'a'}, {66, 'b'}, {67, 'c'}, {68, 'd'} }; cout << "Value of key ASCII 66 = " << ASCII.at(66) << endl; return 0; }
Output
Output of the above code is as follows −
Value of key ASCII 66 = b