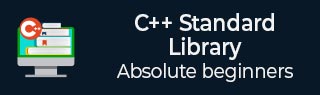
- The C Standard Library
- The C Standard Library
- The C++ Standard Library
- C++ Library - Home
- C++ Library - <fstream>
- C++ Library - <iomanip>
- C++ Library - <ios>
- C++ Library - <iosfwd>
- C++ Library - <iostream>
- C++ Library - <istream>
- C++ Library - <ostream>
- C++ Library - <sstream>
- C++ Library - <streambuf>
- C++ Library - <atomic>
- C++ Library - <complex>
- C++ Library - <exception>
- C++ Library - <functional>
- C++ Library - <limits>
- C++ Library - <locale>
- C++ Library - <memory>
- C++ Library - <new>
- C++ Library - <numeric>
- C++ Library - <regex>
- C++ Library - <stdexcept>
- C++ Library - <string>
- C++ Library - <thread>
- C++ Library - <tuple>
- C++ Library - <typeinfo>
- C++ Library - <utility>
- C++ Library - <valarray>
- The C++ STL Library
- C++ Library - <array>
- C++ Library - <bitset>
- C++ Library - <deque>
- C++ Library - <forward_list>
- C++ Library - <list>
- C++ Library - <map>
- C++ Library - <queue>
- C++ Library - <set>
- C++ Library - <stack>
- C++ Library - <unordered_map>
- C++ Library - <unordered_set>
- C++ Library - <vector>
- C++ Library - <algorithm>
- C++ Library - <iterator>
- C++ Programming Resources
- C++ Programming Tutorial
- C++ Useful Resources
- C++ Discussion
C++ Tuple Library - swap
Description
It exchanges the content of the tuple object by the content of tpl, which is another tuple of the same type.
Declaration
Following is the declaration for std::tuple::swap.
C++98
void swap (tuple& tpl) noexcept
C++11
void swap (tuple& tpl) noexcept
Parameters
tpl − It is an another tuple object of the same type.
Return Value
none
Exceptions
No-throw guarantee − this member function never throws exceptions.
Data races
The members of both tuple objects are modified.
Example
In below example for std::tuple::swap.
#include <iostream> #include <tuple> int main () { std::tuple<int,char> a (50,'a'); std::tuple<int,char> b (200,'b'); a.swap(b); std::cout << "a contains: " << std::get<0>(a); std::cout << " and " << std::get<1>(a) << '\n'; return 0; }
The output should be like this −
a contains: 200 and b
tuple.htm
Advertisements
To Continue Learning Please Login