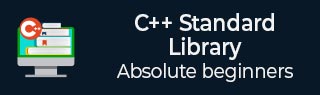
- The C Standard Library
- The C Standard Library
- The C++ Standard Library
- C++ Library - Home
- C++ Library - <fstream>
- C++ Library - <iomanip>
- C++ Library - <ios>
- C++ Library - <iosfwd>
- C++ Library - <iostream>
- C++ Library - <istream>
- C++ Library - <ostream>
- C++ Library - <sstream>
- C++ Library - <streambuf>
- C++ Library - <atomic>
- C++ Library - <complex>
- C++ Library - <exception>
- C++ Library - <functional>
- C++ Library - <limits>
- C++ Library - <locale>
- C++ Library - <memory>
- C++ Library - <new>
- C++ Library - <numeric>
- C++ Library - <regex>
- C++ Library - <stdexcept>
- C++ Library - <string>
- C++ Library - <thread>
- C++ Library - <tuple>
- C++ Library - <typeinfo>
- C++ Library - <utility>
- C++ Library - <valarray>
- The C++ STL Library
- C++ Library - <array>
- C++ Library - <bitset>
- C++ Library - <deque>
- C++ Library - <forward_list>
- C++ Library - <list>
- C++ Library - <map>
- C++ Library - <multimap>
- C++ Library - <queue>
- C++ Library - <priority_queue>
- C++ Library - <set>
- C++ Library - <stack>
- C++ Library - <unordered_map>
- C++ Library - <unordered_set>
- C++ Library - <vector>
- C++ Library - <algorithm>
- C++ Library - <iterator>
- C++ Programming Resources
- C++ Programming Tutorial
- C++ Useful Resources
- C++ Discussion
C++ Tuple::constructor
The C++ std::tuple::constructor is used to initialize the tuple object grouping multiple elements into single entity. It provides a convenient way for the creation of the tuples. It accepts arguments corresponding to the types and number of elements in tuple,constructing it accordingly.
Tuples can hold elements of different data types, for flexible manipulation and data storage.
Syntax
Following is the syntax for std::tuple::constructor.
constexpr tuple();
Parameters
- It is default constructor, that constructs a tuple object with its elements value-initialized.
Return Value
This function does not return anything.
Example
Let's look at the following example, where we are going to create a tuple from individual values.
#include <iostream> #include <tuple> int main() { std::tuple<int, char, double> x(1, 'B', 2.3); std::cout << "The Elements Are : " << std::get<0>(x) << ", " << std::get<1>(x) << ", " << std::get<2>(x) << std::endl; return 0; }
Output
Let us compile and run the above program, this will produce the following result −
The Elements Are : 1, B, 2.3
Example
Consider the another scenario, where we are going to construct a tuple using another tuple.
#include <iostream> #include <tuple> int main() { std::tuple<int, std::string> x(1, "TutorialsPoint"); std::tuple<int, std::string> y(x); std::cout << std::get<0>(y) << std::endl; std::cout << std::get<1>(y) << std::endl; return 0; }
Output
If we run the above code it will generate the following output −
4 TutorialsPoint
Example
In the following example, we are going to construct the tuple using the initializer list.
#include <iostream> #include <tuple> int main() { auto x = std::make_tuple(1, 3.14); std::cout << std::get<0>(x) << std::endl; std::cout << std::get<1>(x) << std::endl; return 0; }
Output
Following is the output of the above code −
1 3.14
Example
Following is the example, where we are going to construct the tuple by explicitly.
#include <iostream> #include <tuple> int main() { std::tuple<int, std::string> x = std::make_tuple<int, std::string>(1, "TutorialsPoint"); std::cout << std::get<0>(x) << std::endl; std::cout << std::get<1>(x) << std::endl; return 0; }
Output
Output of the above code is as follows −
1 TutorialsPoint