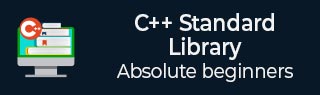
- The C Standard Library
- The C Standard Library
- The C++ Standard Library
- C++ Library - Home
- C++ Library - <fstream>
- C++ Library - <iomanip>
- C++ Library - <ios>
- C++ Library - <iosfwd>
- C++ Library - <iostream>
- C++ Library - <istream>
- C++ Library - <ostream>
- C++ Library - <sstream>
- C++ Library - <streambuf>
- C++ Library - <atomic>
- C++ Library - <complex>
- C++ Library - <exception>
- C++ Library - <functional>
- C++ Library - <limits>
- C++ Library - <locale>
- C++ Library - <memory>
- C++ Library - <new>
- C++ Library - <numeric>
- C++ Library - <regex>
- C++ Library - <stdexcept>
- C++ Library - <string>
- C++ Library - <thread>
- C++ Library - <tuple>
- C++ Library - <typeinfo>
- C++ Library - <utility>
- C++ Library - <valarray>
- The C++ STL Library
- C++ Library - <array>
- C++ Library - <bitset>
- C++ Library - <deque>
- C++ Library - <forward_list>
- C++ Library - <list>
- C++ Library - <map>
- C++ Library - <queue>
- C++ Library - <set>
- C++ Library - <stack>
- C++ Library - <unordered_map>
- C++ Library - <unordered_set>
- C++ Library - <vector>
- C++ Library - <algorithm>
- C++ Library - <iterator>
- C++ Programming Resources
- C++ Programming Tutorial
- C++ Useful Resources
- C++ Discussion
C++ Tuple Library - tuple_cat
Description
It constructs an object of the appropriate tuple type to contain a concatenation of the elements of all the tuples in tpls, in the same order.
Declaration
Following is the declaration for std::tuple_cat.
C++98
template <class... Tuples> tuple<CTypes...> tuple_cat (Tuples&&... tpls);
C++11
template <class... Tuples> tuple<CTypes...> tuple_cat (Tuples&&... tpls);
C++14
template <class... Tuples> tuple<CTypes...> tuple_cat (Tuples&&... tpls);
Parameters
tpls − It separats list of tuple objects. These may be of different types.
Return Value
It returns a tuple object of the appropriate type to hold args.
Exceptions
No-throw guarantee − this member function never throws exceptions.
Data races
None introduced by this call.
Example
In below example for std::tuple_cat.
#include <iostream> #include <utility> #include <string> #include <tuple> int main () { std::tuple<float,std::string> mytuple (3.14,"pi"); std::pair<int,char> mypair (100,'x'); auto myauto = std::tuple_cat ( mytuple, std::tuple<int,char>(mypair) ); std::cout << "myauto contains: " << '\n'; std::cout << std::get<0>(myauto) << '\n'; std::cout << std::get<1>(myauto) << '\n'; std::cout << std::get<2>(myauto) << '\n'; std::cout << std::get<3>(myauto) << '\n'; return 0; }
The output should be like this −
myauto contains: 3.14 pi 100 x
tuple.htm
Advertisements