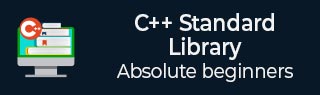
- The C Standard Library
- The C Standard Library
- The C++ Standard Library
- C++ Library - Home
- C++ Library - <fstream>
- C++ Library - <iomanip>
- C++ Library - <ios>
- C++ Library - <iosfwd>
- C++ Library - <iostream>
- C++ Library - <istream>
- C++ Library - <ostream>
- C++ Library - <sstream>
- C++ Library - <streambuf>
- C++ Library - <atomic>
- C++ Library - <complex>
- C++ Library - <exception>
- C++ Library - <functional>
- C++ Library - <limits>
- C++ Library - <locale>
- C++ Library - <memory>
- C++ Library - <new>
- C++ Library - <numeric>
- C++ Library - <regex>
- C++ Library - <stdexcept>
- C++ Library - <string>
- C++ Library - <thread>
- C++ Library - <tuple>
- C++ Library - <typeinfo>
- C++ Library - <utility>
- C++ Library - <valarray>
- The C++ STL Library
- C++ Library - <array>
- C++ Library - <bitset>
- C++ Library - <deque>
- C++ Library - <forward_list>
- C++ Library - <list>
- C++ Library - <map>
- C++ Library - <queue>
- C++ Library - <set>
- C++ Library - <stack>
- C++ Library - <unordered_map>
- C++ Library - <unordered_set>
- C++ Library - <vector>
- C++ Library - <algorithm>
- C++ Library - <iterator>
- C++ Programming Resources
- C++ Programming Tutorial
- C++ Useful Resources
- C++ Discussion
C++ Tuple Library - tie
Description
It constructs a tuple object whose elements are references to the arguments in args, in the same order.
Declaration
Following is the declaration for std::tie.
C++98
template<class... Types> tuple<Types&...> tie (Types&... args) noexcept;
C++11
template<class... Types> tuple<Types&...> tie (Types&... args) noexcept;
C++14
template<class... Types> constexpr tuple<Types&...> tie (Types&... args) noexcept;
Parameters
args − It contains list of elements that the constructed tuple shall contain.
Return Value
It returns a tuple with lvalue references to args.
Exceptions
No-throw guarantee − this member function never throws exceptions.
Data races
None introduced by this call.
Example
In below example for std::tie.
#include <iostream> #include <tuple> int main () { int myint; char mychar; std::tuple<int,float,char> mytuple; mytuple = std::make_tuple (10, 2.6, 'a'); std::tie (myint, std::ignore, mychar) = mytuple; std::cout << "myint contains: " << myint << '\n'; std::cout << "mychar contains: " << mychar << '\n'; return 0; }
The output should be like this −
myint contains: 10 mychar contains: a
tuple.htm
Advertisements