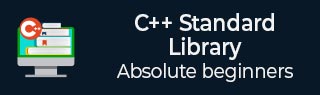
- The C Standard Library
- The C Standard Library
- The C++ Standard Library
- C++ Library - Home
- C++ Library - <fstream>
- C++ Library - <iomanip>
- C++ Library - <ios>
- C++ Library - <iosfwd>
- C++ Library - <iostream>
- C++ Library - <istream>
- C++ Library - <ostream>
- C++ Library - <sstream>
- C++ Library - <streambuf>
- C++ Library - <atomic>
- C++ Library - <complex>
- C++ Library - <exception>
- C++ Library - <functional>
- C++ Library - <limits>
- C++ Library - <locale>
- C++ Library - <memory>
- C++ Library - <new>
- C++ Library - <numeric>
- C++ Library - <regex>
- C++ Library - <stdexcept>
- C++ Library - <string>
- C++ Library - <thread>
- C++ Library - <tuple>
- C++ Library - <typeinfo>
- C++ Library - <utility>
- C++ Library - <valarray>
- The C++ STL Library
- C++ Library - <array>
- C++ Library - <bitset>
- C++ Library - <deque>
- C++ Library - <forward_list>
- C++ Library - <list>
- C++ Library - <map>
- C++ Library - <multimap>
- C++ Library - <queue>
- C++ Library - <priority_queue>
- C++ Library - <set>
- C++ Library - <stack>
- C++ Library - <unordered_map>
- C++ Library - <unordered_set>
- C++ Library - <vector>
- C++ Library - <algorithm>
- C++ Library - <iterator>
- C++ Programming Resources
- C++ Programming Tutorial
- C++ Useful Resources
- C++ Discussion
C++ Queue::push() Function
The C++ std::queue::push() function is used to insert element to the end of the queue. When this function is invoked it increases the queue size by one and expands. The queue is a FIFO (First-In-First-Out) manner, indicting that the elements are processed in the order they are added.
when the push() function is invoked it adds the specified element at the back of the queue, ensuring that it will be last to be removed when the pop() function is invoked.
Syntax
Following is the syntax for std::queue::push() function.
void push (const value_type& val);
Parameters
- Val − It indicates the value to be assigned to newly inserted element.
Return value
This function does not return anything.
Example
Let's look at the following example, where we are going to demonstrate the usage of push() function.
#include <iostream> #include <queue> int main() { std::queue<int> x; x.push(1); x.push(222); std::cout << "Queue Size: " << x.size() << std::endl; return 0; }
Output
Output of the above code is as follows −
Queue Size: 2
Example
Consider the following example, where we are going to add strings to the queue and accessing the front element using front() function.
#include <iostream> #include <queue> #include <string> int main() { std::queue<std::string> x; x.push("TutorialsPoint"); x.push("TP"); std::cout << "Front Element: " << x.front() << std::endl; return 0; }
Output
Let us compile and run the above program, this will produce the following result −
Front Element: TutorialsPoint
Example
In the following example, we are going to add the elements into the queue using the loop.
#include <iostream> #include <queue> int main() { std::queue<int> a; for (int x = 0; x < 3; ++x) { a.push(x * 3); } std::cout << "Queue Size: " << a.size() << std::endl; return 0; }
Output
If we run the above code it will generate the following output −
Queue Size: 3
Example
Following is the example, where we are going to push the elements from an array into the queue.
#include <iostream> #include <queue> int main() { int array[] = {1, 2, 3, 4, 5}; std::queue<int> a; for(int x = 0; x < 4; ++x) { a.push(array[x]); } std::cout << "Queue size: " << a.size() << std::endl; return 0; }
Output
Output of the above code is as follows −
Queue size: 4