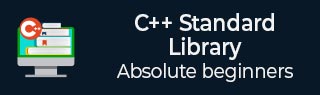
- The C Standard Library
- The C Standard Library
- The C++ Standard Library
- C++ Library - Home
- C++ Library - <fstream>
- C++ Library - <iomanip>
- C++ Library - <ios>
- C++ Library - <iosfwd>
- C++ Library - <iostream>
- C++ Library - <istream>
- C++ Library - <ostream>
- C++ Library - <sstream>
- C++ Library - <streambuf>
- C++ Library - <atomic>
- C++ Library - <complex>
- C++ Library - <exception>
- C++ Library - <functional>
- C++ Library - <limits>
- C++ Library - <locale>
- C++ Library - <memory>
- C++ Library - <new>
- C++ Library - <numeric>
- C++ Library - <regex>
- C++ Library - <stdexcept>
- C++ Library - <string>
- C++ Library - <thread>
- C++ Library - <tuple>
- C++ Library - <typeinfo>
- C++ Library - <utility>
- C++ Library - <valarray>
- The C++ STL Library
- C++ Library - <array>
- C++ Library - <bitset>
- C++ Library - <deque>
- C++ Library - <forward_list>
- C++ Library - <list>
- C++ Library - <map>
- C++ Library - <multimap>
- C++ Library - <queue>
- C++ Library - <priority_queue>
- C++ Library - <set>
- C++ Library - <stack>
- C++ Library - <unordered_map>
- C++ Library - <unordered_set>
- C++ Library - <vector>
- C++ Library - <algorithm>
- C++ Library - <iterator>
- C++ Programming Resources
- C++ Programming Tutorial
- C++ Useful Resources
- C++ Discussion
C++ Queue::operator>() Function
The C++ std::queue::operator> function is a relational operator used to compare the objects of the queue. It checks if the first queue is lexicographically greater than the second queue and returns a boolean value true if the element from the first queue is greater than the second queue, otherwise false. The time complexity of this function is linear i.e.O(n).
Syntax
Following is the syntax for std::queue::operator> function.
bool operator> (const queue<T,Container>& q1, const queue<T,Container>& q2);
Parameters
- q1 − It indicates the first queue object.
- q2 − It indicates the second queue object.
Return value
This function returns true if first queue is greater than second otherwise false.
Example
Let's look at the following example, where we are going to initialize a queues with integers and compare them.
#include <iostream> #include <queue> int main() { std::queue<int> a; std::queue<int> b; a.push(1); a.push(22); b.push(1); b.push(2); if (a > b) { std::cout << "Queue1 is greater than Queue2." << std::endl; } else { std::cout << "Queue1 is not greater than Queue2." << std::endl; } return 0; }
Output
Output of the above code is as follows −
Queue1 is greater than Queue2.
Example
Consider the another scenario, where we are going to initialize a queue with characters and apply the operator> function.
#include <iostream> #include <queue> int main() { std::queue<char> a; std::queue<char> b; a.push('a'); a.push('b'); b.push('a'); b.push('c'); if (a > b) { std::cout << "Queue1 is greater than Queue2." << std::endl; } else { std::cout << "Queue1 is not greater than Queue2." << std::endl; } return 0; }
Output
Following is the output of the above code −
Queue1 is not greater than Queue2.
Example
In the following example, we are going to apply the operator> function on the queue that contains float values.
#include <iostream> #include <queue> int main() { std::queue<float> a; std::queue<float> b; a.push(1.1); a.push(0.4); b.push(0.2); b.push(0.5); if (a > b) { std::cout << "Queue1 is greater than Queue2." << std::endl; } else { std::cout << "Queue1 is not greater than Queue2." << std::endl; } return 0; }
Output
Let us compile and run the above program, this will produce the following result −
Queue1 is greater than Queue2.
Example
Following is the example, where we are going to apply the operator> function on the empty queue and observing the output.
#include <iostream> #include <queue> int main() { std::queue<int> a; std::queue<int> b; if (a > b) { std::cout << "Queue1 is greater than Queue2." << std::endl; } else { std::cout << "Queue1 is not greater than Queue2." << std::endl; } return 0; }
Output
If we run the above code it will generate the following output −
Queue1 is not greater than Queue2.