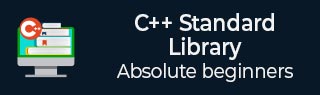
- The C Standard Library
- The C Standard Library
- The C++ Standard Library
- C++ Library - Home
- C++ Library - <fstream>
- C++ Library - <iomanip>
- C++ Library - <ios>
- C++ Library - <iosfwd>
- C++ Library - <iostream>
- C++ Library - <istream>
- C++ Library - <ostream>
- C++ Library - <sstream>
- C++ Library - <streambuf>
- C++ Library - <atomic>
- C++ Library - <complex>
- C++ Library - <exception>
- C++ Library - <functional>
- C++ Library - <limits>
- C++ Library - <locale>
- C++ Library - <memory>
- C++ Library - <new>
- C++ Library - <numeric>
- C++ Library - <regex>
- C++ Library - <stdexcept>
- C++ Library - <string>
- C++ Library - <thread>
- C++ Library - <tuple>
- C++ Library - <typeinfo>
- C++ Library - <utility>
- C++ Library - <valarray>
- The C++ STL Library
- C++ Library - <array>
- C++ Library - <bitset>
- C++ Library - <deque>
- C++ Library - <forward_list>
- C++ Library - <list>
- C++ Library - <map>
- C++ Library - <multimap>
- C++ Library - <queue>
- C++ Library - <priority_queue>
- C++ Library - <set>
- C++ Library - <stack>
- C++ Library - <unordered_map>
- C++ Library - <unordered_set>
- C++ Library - <vector>
- C++ Library - <algorithm>
- C++ Library - <iterator>
- C++ Programming Resources
- C++ Programming Tutorial
- C++ Useful Resources
- C++ Discussion
C++ priority_queue::push() Function
The C++ std::priority_queue::push() function is used to insert the elements into the priority_queue based on the specified comparison. When this function is invoked it adds the provided element to the queue according to its priority, that is determined by the comparison function. The new element is placed at a position, where the queue maintains its order, ensuring that the highest priority elements remains at the front.
Syntax
Following is the syntax for std::priority_queue::push() function.
void push (const value_type& val); or void push (value_type&& val);
Parameters
- val − It indicates the value to be assigned to newly inserted element.
Return value
This function does not return anything.
Example
Let's look at the following example, where we are going to demonstrate the usage of push() function.
#include <iostream> #include <queue> int main() { std::priority_queue<int> x; x.push(1); x.push(22); x.push(333); while (!x.empty()) { std::cout << x.top() << " "; x.pop(); } return 0; }
Output
Following is the output of the above code −
333 22 1
Example
Consider the following example, where we are going to use the push() function with pairs and retrieving the output.
#include <iostream> #include <queue> #include <vector> int main() { std::priority_queue<std::pair<int, std::string>> a; a.push(std::make_pair(1, "Namaste")); a.push(std::make_pair(2, "Hello")); a.push(std::make_pair(3, "Hi")); while (!a.empty()) { std::cout << a.top().second << " "; a.pop(); } return 0; }
Output
Output of the above code is as follows −
Hi Hello Namaste
Example
In the following example, we are going to use the priority_queue with a custom class.
#include <iostream> #include <queue> #include <vector> struct x { int priority; std::string description; bool operator<(const x& other) const { return priority < other.priority; } }; int main() { std::priority_queue<x> a; a.push(x{3, "TutorialsPoint"}); a.push(x{2, "Tutorix"}); a.push(x{1, "TP"}); std::cout << " " << a.top().description << std::endl; return 0; }
Output
If we run the above code it will generate the following output −
TutorialsPoint