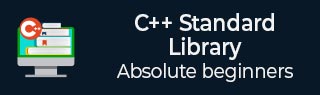
- The C Standard Library
- The C Standard Library
- The C++ Standard Library
- C++ Library - Home
- C++ Library - <fstream>
- C++ Library - <iomanip>
- C++ Library - <ios>
- C++ Library - <iosfwd>
- C++ Library - <iostream>
- C++ Library - <istream>
- C++ Library - <ostream>
- C++ Library - <sstream>
- C++ Library - <streambuf>
- C++ Library - <atomic>
- C++ Library - <complex>
- C++ Library - <exception>
- C++ Library - <functional>
- C++ Library - <limits>
- C++ Library - <locale>
- C++ Library - <memory>
- C++ Library - <new>
- C++ Library - <numeric>
- C++ Library - <regex>
- C++ Library - <stdexcept>
- C++ Library - <string>
- C++ Library - <thread>
- C++ Library - <tuple>
- C++ Library - <typeinfo>
- C++ Library - <utility>
- C++ Library - <valarray>
- The C++ STL Library
- C++ Library - <array>
- C++ Library - <bitset>
- C++ Library - <deque>
- C++ Library - <forward_list>
- C++ Library - <list>
- C++ Library - <map>
- C++ Library - <multimap>
- C++ Library - <queue>
- C++ Library - <priority_queue>
- C++ Library - <set>
- C++ Library - <stack>
- C++ Library - <unordered_map>
- C++ Library - <unordered_set>
- C++ Library - <vector>
- C++ Library - <algorithm>
- C++ Library - <iterator>
- C++ Programming Resources
- C++ Programming Tutorial
- C++ Useful Resources
- C++ Discussion
C++ priority_queue::operator=() Function
The C++ std::priority_queue::operator=() function is used to assign the content of one priority_queue to the another. It ensures that the target priority_queue becomes the exact copy of the source, with the same elements and ordering.
It handles both copy and move semantics, providing the efficient operations depending on whether the sources is an l-value(copy) or an r-value(move) you can find the syntaxes of both the variants below. The time complexity of this function is linear i.e.O(n).
Syntax
Following is the syntax for std::priority_queue::operator=() function.
operator=( const priority_queue<T,Container>& other ); or operator=( priority_queue<T,Container>&& other );
Parameters
- other − It indicates the another priority_queue objecct of same type.
Return value
This function returns this pointer.
Example
In the following example, we are going to demonstrate the basic usage of the assignment operator.
#include <iostream> #include <queue> #include <vector> int main() { std::priority_queue<int> a; a.push(1); a.push(2); std::priority_queue<int> b; b.push(3); b.push(4); b = a; while (!b.empty()) { std::cout << b.top() << " "; b.pop(); } return 0; }
Output
Output of the above code is as follows −
2 1
Example
Consider the following example, where we are going to assign a priority_queue to itself.
#include <iostream> #include <queue> int main() { std::priority_queue<int> a; a.push(1); a.push(2); a.push(3); a = a; while (!a.empty()) { std::cout << a.top() << " "; a.pop(); } return 0; }
Output
If we run the above code it will generate the following output −
3 2 1
Example
In the following example, we are going to assign an initially empty priority_queue to another priority_queue using the assignment operator.
#include <iostream> #include <queue> int main() { std::priority_queue<int> a; std::priority_queue<int> b; b.push(20); b.push(30); b = a; std::cout << "Size of the queue2 after assigning: " << b.size(); return 0; }
Output
Let us compile and run the above program, this will produce the following result −
Size of the queue2 after assigning: 0