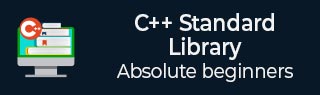
- The C Standard Library
- The C Standard Library
- The C++ Standard Library
- C++ Library - Home
- C++ Library - <fstream>
- C++ Library - <iomanip>
- C++ Library - <ios>
- C++ Library - <iosfwd>
- C++ Library - <iostream>
- C++ Library - <istream>
- C++ Library - <ostream>
- C++ Library - <sstream>
- C++ Library - <streambuf>
- C++ Library - <atomic>
- C++ Library - <complex>
- C++ Library - <exception>
- C++ Library - <functional>
- C++ Library - <limits>
- C++ Library - <locale>
- C++ Library - <memory>
- C++ Library - <new>
- C++ Library - <numeric>
- C++ Library - <regex>
- C++ Library - <stdexcept>
- C++ Library - <string>
- C++ Library - <thread>
- C++ Library - <tuple>
- C++ Library - <typeinfo>
- C++ Library - <utility>
- C++ Library - <valarray>
- The C++ STL Library
- C++ Library - <array>
- C++ Library - <bitset>
- C++ Library - <deque>
- C++ Library - <forward_list>
- C++ Library - <list>
- C++ Library - <map>
- C++ Library - <multimap>
- C++ Library - <queue>
- C++ Library - <priority_queue>
- C++ Library - <set>
- C++ Library - <stack>
- C++ Library - <unordered_map>
- C++ Library - <unordered_set>
- C++ Library - <vector>
- C++ Library - <algorithm>
- C++ Library - <iterator>
- C++ Programming Resources
- C++ Programming Tutorial
- C++ Useful Resources
- C++ Discussion
C++ New::operator new[]
The C++ New::operator new[] returns a pointer to the first element after allocating and initialising an array of objects. In contrast, the new operator behaves the same way but only allows for a single allocation rather than an array. Use new with delete and new[] with delete[] for deallocation and object deletion.
Although the operator new[] can be invoked explicitly like any other function in C++, it has a specific behavior. If an expression uses the new operator on an array type, it first calls the function operator new with the array type specifier's size as the first parameter. If this is successful, it then automatically initializes or builds each object in the array.
Syntax
Following is the syntax for C++ New::operator new[] −
void* operator new[] (std::size_t size) throw (std::bad_alloc); (throwing allocation) void* operator new[] (std::size_t size, const std::nothrow_t& nothrow_value) throw(); (nothrow allocation) void* operator new[] (std::size_t size, void* ptr) throw(); (placement)
Parameters
- size − It contain size in bytes of the requested memory block.
- nothrow_value − It contains the constant nothrow.
- ptr − It is a pointer to an already-allocated memory block of the proper size.
Example 1
Let's look into the following example, where we are going to use the operator new[] and retriveing the output.
#include <cstdio> #include <cstdlib> #include <new> void* operator new[](std::size_t sz) { std::printf(" operator new[](size_t), size = %zu\n", sz); if (sz == 1) ++sz; if (void *ptr = std::malloc(sz)) return ptr; throw std::bad_alloc{}; } int main() { int* p2 = new int[12]; delete[] p2; }
Output
Let us compile and run the above program, this will produce the following result −
operator new[](size_t), size = 48
Example 2
Let's look into the another scenario, where we are going to use the operator new[] along with the specific allocations.
#include <iostream> struct A { static void* operator new(std::size_t count) { std::cout << "custom operator new for size " << count << '\n'; return ::operator new(count); } static void* operator new[](std::size_t count) { std::cout << "custom operator new[] for size " << count << '\n'; return ::operator new[](count); } }; int main() { A* p1 = new A; delete p1; A* p2 = new A[13]; delete[] p2; }
Output
On running the above code, it will display the output as shown below −
custom operator new for size 1 custom operator new[] for size 13
Example 3
Considering the following where we are going to check the functioning of operator new[].
#include <iostream> #include <new> struct MyClass { int data; MyClass() { std::cout << '@'; } }; int main () { std::cout << "constructions (1): "; MyClass * p1 = new MyClass[10]; std::cout << '\n'; std::cout << "constructions (2): "; MyClass * p2 = new (std::nothrow) MyClass[5]; std::cout << '\n'; delete[] p2; delete[] p1; return 0; }
Output
when the code gets executed, it will generate the output as shown below −
constructions (1): @@@@@@@@@@ constructions (2): @@@@@