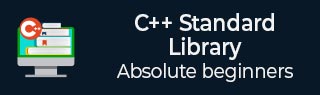
- The C Standard Library
- The C Standard Library
- The C++ Standard Library
- C++ Library - Home
- C++ Library - <fstream>
- C++ Library - <iomanip>
- C++ Library - <ios>
- C++ Library - <iosfwd>
- C++ Library - <iostream>
- C++ Library - <istream>
- C++ Library - <ostream>
- C++ Library - <sstream>
- C++ Library - <streambuf>
- C++ Library - <atomic>
- C++ Library - <complex>
- C++ Library - <exception>
- C++ Library - <functional>
- C++ Library - <limits>
- C++ Library - <locale>
- C++ Library - <memory>
- C++ Library - <new>
- C++ Library - <numeric>
- C++ Library - <regex>
- C++ Library - <stdexcept>
- C++ Library - <string>
- C++ Library - <thread>
- C++ Library - <tuple>
- C++ Library - <typeinfo>
- C++ Library - <utility>
- C++ Library - <valarray>
- The C++ STL Library
- C++ Library - <array>
- C++ Library - <bitset>
- C++ Library - <deque>
- C++ Library - <forward_list>
- C++ Library - <list>
- C++ Library - <map>
- C++ Library - <queue>
- C++ Library - <set>
- C++ Library - <stack>
- C++ Library - <unordered_map>
- C++ Library - <unordered_set>
- C++ Library - <vector>
- C++ Library - <algorithm>
- C++ Library - <iterator>
- C++ Programming Resources
- C++ Programming Tutorial
- C++ Useful Resources
- C++ Discussion
C++ Map Library - operator< Function
Description
The C++ function std::multimap::operator< tests whether first multimap is less than other or not.
Operator < compares element sequentially and comparison stops at first mismatch.
Declaration
Following is the declaration for std::multimap::operator< function form std::map header.
C++98
template <class Key, class T, class Compare, class Alloc> bool operator< ( const multimap<Key,T,Compare,Alloc>& m1, const multimap<Key,T,Compare,Alloc>& m2);
Parameters
m1 − First multimap object.
m2 − Second multimap object.
Return value
Returns true if first multimap is less than second otherwise false.
Exceptions
No effect on container if exception is thrown.
Time complexity
Linear i.e. O(n)
Example
The following example shows the usage of std::multimap::operator< function.
#include <iostream> #include <map> using namespace std; int main(void) { /* Multimap with duplicates */ multimap<char, int> m1; multimap<char, int> m2; m2.insert(pair<char, int>('a', 1)); if (m1 < m2) cout << "m1 multimap is less than m2." << endl; m1 = m2; if (!(m1 < m2)) cout << "m1 multimap is not less than m2." << endl; return 0; }
Let us compile and run the above program, this will produce the following result −
m1 multimap is less than m2. m1 multimap is not less than m2.
map.htm
Advertisements