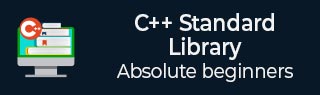
- The C Standard Library
- The C Standard Library
- The C++ Standard Library
- C++ Library - Home
- C++ Library - <fstream>
- C++ Library - <iomanip>
- C++ Library - <ios>
- C++ Library - <iosfwd>
- C++ Library - <iostream>
- C++ Library - <istream>
- C++ Library - <ostream>
- C++ Library - <sstream>
- C++ Library - <streambuf>
- C++ Library - <atomic>
- C++ Library - <complex>
- C++ Library - <exception>
- C++ Library - <functional>
- C++ Library - <limits>
- C++ Library - <locale>
- C++ Library - <memory>
- C++ Library - <new>
- C++ Library - <numeric>
- C++ Library - <regex>
- C++ Library - <stdexcept>
- C++ Library - <string>
- C++ Library - <thread>
- C++ Library - <tuple>
- C++ Library - <typeinfo>
- C++ Library - <utility>
- C++ Library - <valarray>
- The C++ STL Library
- C++ Library - <array>
- C++ Library - <bitset>
- C++ Library - <deque>
- C++ Library - <forward_list>
- C++ Library - <list>
- C++ Library - <map>
- C++ Library - <multimap>
- C++ Library - <queue>
- C++ Library - <priority_queue>
- C++ Library - <set>
- C++ Library - <stack>
- C++ Library - <unordered_map>
- C++ Library - <unordered_set>
- C++ Library - <vector>
- C++ Library - <algorithm>
- C++ Library - <iterator>
- C++ Programming Resources
- C++ Programming Tutorial
- C++ Useful Resources
- C++ Discussion
C++ multimap::operator>=() Function
The C++ std::multimap::operator>=() function is used to compare two multimaps to determine whether the first multimap is lexicographically greater than or equal to the second multimap. This comparison involves the keys and values in the multimap, proceeding from the beginning to the end. It return true if all corresponding elements in the first multimap is greater than or equal to those in the second multimap.
Syntax
Following is the syntax for std::multimap::operator>=() function.
bool operator>=( const std::multimap<Key, T, Compare, Alloc>& lhs, const std::multimap<Key, T, Compare, Alloc>& rhs );
Parameters
- lhs − It indicates the first multimap object.
- rhs − It indicates the second multimap object.
Return value
This function returns true if first multimap is greater than or equal to second otherwise false.
Exceptions
It does not make any changes on container if exception is thrown.
Time complexity
The time complexity of this function is Linear i.e. O(n)
Example
Let's look at the following example, where we are going to demonstrate the usage of the operator >=() function.
#include <iostream> #include <map> using namespace std; int main(void) { multimap<char, int> m1; multimap<char, int> m2; m1.insert(pair<char, int>('a', 1)); if (m1 >= m2) cout << "m1 multimap is greater than or equal to m2." << endl; m2.insert(pair<char, int>('a', 1)); m2.insert(pair<char, int>('a', 1)); if (!(m1 >= m2)) cout << "m1 multimap is not greater than or equal to m2." << endl; return 0; }
Output
Output of the above code is as follows −
m1 multimap is greater than or equal to m2. m1 multimap is not greater than or equal to m2.