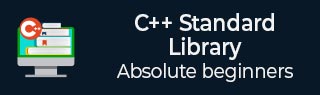
- The C Standard Library
- The C Standard Library
- The C++ Standard Library
- C++ Library - Home
- C++ Library - <fstream>
- C++ Library - <iomanip>
- C++ Library - <ios>
- C++ Library - <iosfwd>
- C++ Library - <iostream>
- C++ Library - <istream>
- C++ Library - <ostream>
- C++ Library - <sstream>
- C++ Library - <streambuf>
- C++ Library - <atomic>
- C++ Library - <complex>
- C++ Library - <exception>
- C++ Library - <functional>
- C++ Library - <limits>
- C++ Library - <locale>
- C++ Library - <memory>
- C++ Library - <new>
- C++ Library - <numeric>
- C++ Library - <regex>
- C++ Library - <stdexcept>
- C++ Library - <string>
- C++ Library - <thread>
- C++ Library - <tuple>
- C++ Library - <typeinfo>
- C++ Library - <utility>
- C++ Library - <valarray>
- The C++ STL Library
- C++ Library - <array>
- C++ Library - <bitset>
- C++ Library - <deque>
- C++ Library - <forward_list>
- C++ Library - <list>
- C++ Library - <map>
- C++ Library - <multimap>
- C++ Library - <queue>
- C++ Library - <priority_queue>
- C++ Library - <set>
- C++ Library - <stack>
- C++ Library - <unordered_map>
- C++ Library - <unordered_set>
- C++ Library - <vector>
- C++ Library - <algorithm>
- C++ Library - <iterator>
- C++ Programming Resources
- C++ Programming Tutorial
- C++ Useful Resources
- C++ Discussion
C++ Map Library - operator= Function
Description
The C++ function std::multimap::operator= move the contents of one multimap into another and modifies size if necessary.
Declaration
Following is the declaration for std::multimap::operator= function form std::map header.
C++11
multimap& operator= (multimap&& x);
Parameters
x − Another multimap object of same type.
Return value
Returns this pointer
Exceptions
No effect on container if exception is thrown.
Time complexity
Linear i.e. O(n)
Example
The following example shows the usage of std::multimap::operator= function.
#include <iostream> #include <map> using namespace std; int main(void) { /* Multimap with duplicates */ multimap<char, int> m1 { {'a', 1}, {'a', 2}, {'b', 3}, {'c', 4}, {'d', 5} }; multimap<char, int> m2; m2 = move(m1); cout << "Multimap contains following elements" << endl; for (auto it = m2.begin(); it != m2.end(); ++it) cout << it->first << " = " << it->second << endl; return 0; }
Let us compile and run the above program, this will produce the following result −
Multimap contains following elements a = 1 a = 2 b = 3 c = 4 d = 5
map.htm
Advertisements