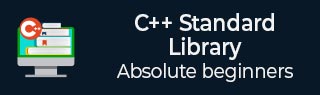
- The C Standard Library
- The C Standard Library
- The C++ Standard Library
- C++ Library - Home
- C++ Library - <fstream>
- C++ Library - <iomanip>
- C++ Library - <ios>
- C++ Library - <iosfwd>
- C++ Library - <iostream>
- C++ Library - <istream>
- C++ Library - <ostream>
- C++ Library - <sstream>
- C++ Library - <streambuf>
- C++ Library - <atomic>
- C++ Library - <complex>
- C++ Library - <exception>
- C++ Library - <functional>
- C++ Library - <limits>
- C++ Library - <locale>
- C++ Library - <memory>
- C++ Library - <new>
- C++ Library - <numeric>
- C++ Library - <regex>
- C++ Library - <stdexcept>
- C++ Library - <string>
- C++ Library - <thread>
- C++ Library - <tuple>
- C++ Library - <typeinfo>
- C++ Library - <utility>
- C++ Library - <valarray>
- The C++ STL Library
- C++ Library - <array>
- C++ Library - <bitset>
- C++ Library - <deque>
- C++ Library - <forward_list>
- C++ Library - <list>
- C++ Library - <map>
- C++ Library - <multimap>
- C++ Library - <queue>
- C++ Library - <priority_queue>
- C++ Library - <set>
- C++ Library - <stack>
- C++ Library - <unordered_map>
- C++ Library - <unordered_set>
- C++ Library - <vector>
- C++ Library - <algorithm>
- C++ Library - <iterator>
- C++ Programming Resources
- C++ Programming Tutorial
- C++ Useful Resources
- C++ Discussion
C++ multimap::operator=() Function
The C++ std::multimap::operator=() function is used to assigns the contents of one multimap to another, enabling the efficient copying of key value pairs. When this function is invoked, it copies all the elements from the source multimap to the target,maintaining the sorted order.
This function has 3 polymorphic variants: with using the copy version, move version and the initializer list version (you can find the syntaxes of all the variants below).
This function does not merge elements, it replaces the contents of the target multimap with those of the source.
Syntax
Following is the syntax for std::multimap::operator=() function.
multimap& operator= (const multimap& x); or multimap& operator= (multimap&& x); or multimap& operator= (initializer_list<value_type> il);
Parameters
- x − It indicates another multimap object of same type.
- il − It indicates an initializer_list object.
Return value
This function returns this pointer
Example
Let's look at the following example, where we are going to demonstrate the usage of operator=() function.
#include <iostream> #include <map> int main() { std::multimap<int, std::string> a; a.insert({1, "TP"}); a.insert({2, "TutorialsPoint"}); std::multimap<int, std::string> b; b = a; for (const auto& pair : b) { std::cout << pair.first << ": " << pair.second << std::endl; } return 0; }
Output
Output of the above code is as follows −
1: TP 2: TutorialsPoint
Example
Consider the following example, where we are going to make self assignment and observe the output.
#include <iostream> #include <map> int main() { std::multimap<int, std::string> a = {{1, "Hi"}, {1, "Hello"}}; a = a; for (const auto& pair : a) { std::cout << pair.first << " : " << pair.second << std::endl; } return 0; }
Output
Following is the output of the above code −
1 : Hi 1 : Hello
Example
In the following example, we are going to apply the operator=() for assigning the content to the empty multimap.
#include <iostream> #include <map> int main() { std::multimap<int, std::string> a = {{3, "Cruze"}, {2, "Sail"}}; std::multimap<int, std::string> b; b = a; for (const auto& pair : b) { std::cout << pair.first << " : " << pair.second << std::endl; } return 0; }
Output
If we run the above code it will generate the following output −
2 : Sail 3 : Cruze