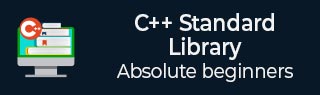
- The C Standard Library
- The C Standard Library
- The C++ Standard Library
- C++ Library - Home
- C++ Library - <fstream>
- C++ Library - <iomanip>
- C++ Library - <ios>
- C++ Library - <iosfwd>
- C++ Library - <iostream>
- C++ Library - <istream>
- C++ Library - <ostream>
- C++ Library - <sstream>
- C++ Library - <streambuf>
- C++ Library - <atomic>
- C++ Library - <complex>
- C++ Library - <exception>
- C++ Library - <functional>
- C++ Library - <limits>
- C++ Library - <locale>
- C++ Library - <memory>
- C++ Library - <new>
- C++ Library - <numeric>
- C++ Library - <regex>
- C++ Library - <stdexcept>
- C++ Library - <string>
- C++ Library - <thread>
- C++ Library - <tuple>
- C++ Library - <typeinfo>
- C++ Library - <utility>
- C++ Library - <valarray>
- The C++ STL Library
- C++ Library - <array>
- C++ Library - <bitset>
- C++ Library - <deque>
- C++ Library - <forward_list>
- C++ Library - <list>
- C++ Library - <map>
- C++ Library - <multimap>
- C++ Library - <queue>
- C++ Library - <priority_queue>
- C++ Library - <set>
- C++ Library - <stack>
- C++ Library - <unordered_map>
- C++ Library - <unordered_set>
- C++ Library - <vector>
- C++ Library - <algorithm>
- C++ Library - <iterator>
- C++ Programming Resources
- C++ Programming Tutorial
- C++ Useful Resources
- C++ Discussion
C++ multimap::max_size() Function
The C++ std::multimap::max_size() function is used to returns the maximum number of elements a multimap container can hold based on available memory. It depends on the system and compiler implementation. It provides an upper limit for the containers capacity, allowing to manage memory efficiently and avoid potential overflow issues. The time complexity of this function is constant i.e. O(1).
Syntax
Following is the syntax for std::multimap::max_size() function.
size_type max_size() const noexcept;
Parameters
It does not accept any parameter.
Return value
This function returns the maximum numbers of elements a multimap can hold.
Example
Let's look at the following example, where we are going to demonstrate the usage of max_size() function.
#include <iostream> #include <map> int main() { std::multimap<int, char> a; std::cout << "Max_size of current multimap : " << a.max_size() << std::endl; return 0; }
Output
Following is the output of the above code −
Max_size of current multimap : 230584300921369395
Example
Consider the following example, where we are going to get the current size and max_sixe of the multimap.
#include <iostream> #include <map> int main() { std::multimap<int, char> a; a.insert({1, 'A'}); a.insert({2, 'B'}); std::cout << "Current_size : " << a.size() << std::endl; std::cout << "Max_size : " << a.max_size() << std::endl; return 0; }
Output
Output of the above code is as follows −
Current_size : 2 Max_size : 230584300921369395
Example
In the following example, we are going to check whether is multimap is empty or not.
#include <iostream> #include <map> int main() { std::multimap<int, std::string> a; if (a.max_size() == 0) { std::cout << "It cannot hold any elements." << std::endl; } else { std::cout << "It can hold elements." << std::endl; } return 0; }
Output
If we run the above code it will generate the following output −
It can hold elements.