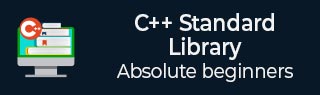
- The C Standard Library
- The C Standard Library
- The C++ Standard Library
- C++ Library - Home
- C++ Library - <fstream>
- C++ Library - <iomanip>
- C++ Library - <ios>
- C++ Library - <iosfwd>
- C++ Library - <iostream>
- C++ Library - <istream>
- C++ Library - <ostream>
- C++ Library - <sstream>
- C++ Library - <streambuf>
- C++ Library - <atomic>
- C++ Library - <complex>
- C++ Library - <exception>
- C++ Library - <functional>
- C++ Library - <limits>
- C++ Library - <locale>
- C++ Library - <memory>
- C++ Library - <new>
- C++ Library - <numeric>
- C++ Library - <regex>
- C++ Library - <stdexcept>
- C++ Library - <string>
- C++ Library - <thread>
- C++ Library - <tuple>
- C++ Library - <typeinfo>
- C++ Library - <utility>
- C++ Library - <valarray>
- The C++ STL Library
- C++ Library - <array>
- C++ Library - <bitset>
- C++ Library - <deque>
- C++ Library - <forward_list>
- C++ Library - <list>
- C++ Library - <map>
- C++ Library - <multimap>
- C++ Library - <queue>
- C++ Library - <priority_queue>
- C++ Library - <set>
- C++ Library - <stack>
- C++ Library - <unordered_map>
- C++ Library - <unordered_set>
- C++ Library - <vector>
- C++ Library - <algorithm>
- C++ Library - <iterator>
- C++ Programming Resources
- C++ Programming Tutorial
- C++ Useful Resources
- C++ Discussion
C++ multimap::lower_bound() Function
The C++ std::multimap::lower_bound() function is used to return an iterator to the first element in the multimap whose key is not less than the specified key (k). This means that it points to the first element that is either equal to or greater than k. If no such element is found, it returns an iterator to the end of the multimap. The time complexity of this function is logarithmic i.e. O(log n).
Syntax
Following is the syntax for std::multimap::lower_bound() function.
iterator lower_bound (const key_type& k); const_iterator lower_bound (const key_type& k) const;
Parameters
- k − It indicates the key to be searched.
Return value
This function returns an iterator to the first element.
Example
Let's look at the following example, where we are going to demonstrate the usage of lower_bound() function.
#include <iostream> #include <map> int main() { std::multimap<int, std::string> a; a.insert({1, "Hi"}); a.insert({2, "Hello"}); a.insert({3, "Vanakam"}); auto x = a.lower_bound(2); if (x != a.end()) { std::cout << "Lower bound key value: " << x->second << std::endl; } return 0; }
Output
Output of the above code is as follows −
Lower bound key value: Hello
Example
Consider the following example, where we are going to apply the lower_bound() on the key that does not exists and observe output.
#include <iostream> #include <map> int main() { std::multimap<int, std::string> a = { {5, "AB"}, {6, "BC"}, {4, "CD"} }; auto x = a.lower_bound(1); if (x != a.end()) { std::cout << "Lower bound key value : (" << x->first << ", " << x->second << ")\n"; } else { std::cout << "Key not found.\n"; } return 0; }
Output
Following is the output of the above code −
Lower bound key value : (4, CD)
Example
In the following example, we are going to use the lower_bound() to get the iterator to the first element and then iterate through the rest of the multimap, printing all elements with keys greater than or equal to lower bound key.
#include <iostream> #include <map> int main() { std::multimap<int, std::string> a = { {1, "Audi"}, {2, "RS5"}, {3, "Cruze"}, {3, "Sail"}, {4, "Beats"} }; auto x = a.lower_bound(3); std::cout << "Elements with lower bound key \n"; for (; x != a.end(); ++x) { std::cout << "(" << x->first << ", " << x->second << ")\n"; } return 0; }
Output
If we run the above code it will generate the following output −
Elements with lower bound key (3, Cruze) (3, Sail) (4, Beats)