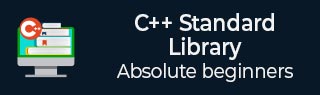
- The C Standard Library
- The C Standard Library
- The C++ Standard Library
- C++ Library - Home
- C++ Library - <fstream>
- C++ Library - <iomanip>
- C++ Library - <ios>
- C++ Library - <iosfwd>
- C++ Library - <iostream>
- C++ Library - <istream>
- C++ Library - <ostream>
- C++ Library - <sstream>
- C++ Library - <streambuf>
- C++ Library - <atomic>
- C++ Library - <complex>
- C++ Library - <exception>
- C++ Library - <functional>
- C++ Library - <limits>
- C++ Library - <locale>
- C++ Library - <memory>
- C++ Library - <new>
- C++ Library - <numeric>
- C++ Library - <regex>
- C++ Library - <stdexcept>
- C++ Library - <string>
- C++ Library - <thread>
- C++ Library - <tuple>
- C++ Library - <typeinfo>
- C++ Library - <utility>
- C++ Library - <valarray>
- The C++ STL Library
- C++ Library - <array>
- C++ Library - <bitset>
- C++ Library - <deque>
- C++ Library - <forward_list>
- C++ Library - <list>
- C++ Library - <map>
- C++ Library - <multimap>
- C++ Library - <queue>
- C++ Library - <priority_queue>
- C++ Library - <set>
- C++ Library - <stack>
- C++ Library - <unordered_map>
- C++ Library - <unordered_set>
- C++ Library - <vector>
- C++ Library - <algorithm>
- C++ Library - <iterator>
- C++ Programming Resources
- C++ Programming Tutorial
- C++ Useful Resources
- C++ Discussion
C++ multimap::empty() Function
The C++ std::multimap::empty() function is used to check whether the multimap is empty or not. It returns a boolean value, true if the multimap is empty, otherwise it return false. This function is helpful for conditional operations where we need to ascertain if the multimap holds any elements before performing further actions. The time complexity of this function is constant i.e.O(1).
Syntax
Following is the syntax for std::multimap::empty() function.
bool empty() const noexcept;
Parameters
It does not accept any parameter.
Return value
This function returns true if multimap is empty otherwise false.
Example
Let's look at the following example, where we are going to demonstrate the usage of empty() function.
#include <iostream> #include <map> int main() { std::multimap<int, std::string> a; if (a.empty()) { std::cout << "Multimap is empty." << std::endl; } else { std::cout << "Multimap is not empty." << std::endl; } return 0; }
Output
Output of the above code is as follows −
Multimap is empty.
Example
Consider the following example, where we are going to add the elements into the multimap and applying the empty() function.
#include <iostream> #include <map> int main() { std::multimap<int, std::string> a; a.insert(std::make_pair(1, "Welcome")); if (a.empty()) { std::cout << "Multimap is empty." << std::endl; } else { std::cout << "Multimap is not empty." << std::endl; } return 0; }
Output
Following is the output of the above code −
Multimap is not empty.
Example
In the following example, we are going to use the empty() function in a loop.
#include <iostream> #include <map> int main() { std::multimap<int, std::string> a; a.insert({1, "Hi"}); while (!a.empty()) { auto x = a.begin(); std::cout << "Removing the element: " << x->second << std::endl; a.erase(x); } if (a.empty()) { std::cout << "Multimap is empty now." << std::endl; } return 0; }
Output
If we run the above code it will generate the following output −
Removing the element: Hi Multimap is empty now.