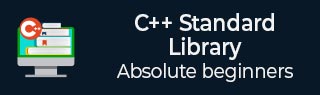
- The C Standard Library
- The C Standard Library
- The C++ Standard Library
- C++ Library - Home
- C++ Library - <fstream>
- C++ Library - <iomanip>
- C++ Library - <ios>
- C++ Library - <iosfwd>
- C++ Library - <iostream>
- C++ Library - <istream>
- C++ Library - <ostream>
- C++ Library - <sstream>
- C++ Library - <streambuf>
- C++ Library - <atomic>
- C++ Library - <complex>
- C++ Library - <exception>
- C++ Library - <functional>
- C++ Library - <limits>
- C++ Library - <locale>
- C++ Library - <memory>
- C++ Library - <new>
- C++ Library - <numeric>
- C++ Library - <regex>
- C++ Library - <stdexcept>
- C++ Library - <string>
- C++ Library - <thread>
- C++ Library - <tuple>
- C++ Library - <typeinfo>
- C++ Library - <utility>
- C++ Library - <valarray>
- The C++ STL Library
- C++ Library - <array>
- C++ Library - <bitset>
- C++ Library - <deque>
- C++ Library - <forward_list>
- C++ Library - <list>
- C++ Library - <map>
- C++ Library - <multimap>
- C++ Library - <queue>
- C++ Library - <priority_queue>
- C++ Library - <set>
- C++ Library - <stack>
- C++ Library - <unordered_map>
- C++ Library - <unordered_set>
- C++ Library - <vector>
- C++ Library - <algorithm>
- C++ Library - <iterator>
- C++ Programming Resources
- C++ Programming Tutorial
- C++ Useful Resources
- C++ Discussion
C++ multimap::crbegin() Function
The C++ std::multimap::crbegin() function is used to return a constant reverse iterator to the last element, enabling read-only traversal from the end to the beginning of the container. This function ensures that the elements are accessed in reverse order without allowing modification. It is mainly useful in the secnarios for read-only operations like accessing and inspecting elements in reverse order. The time complexity of this function is constant i.e.O(1).
Syntax
Following is the syntax for std::multimap::crbegin() function.
const_reverse_iterator crbegin() const noexcept;
Parameters
It does not accept any parameter
Return value
This function returns a constant reverse iterator.
Example
Let's look at the following example, where we are going to demonstrate the basic usage of crbegin() function.
#include <iostream> #include <map> int main() { std::multimap<int, std::string> a = {{1, "Hello"},{3, "Namaste"}, {2, "Hi"}}; auto x = a.crbegin(); std::cout <<"(" << x->first << ", " << x->second << ")\n"; return 0; }
Output
Output of the above code is as follows −
(3, Namaste)
Example
Consider the following example, where we are going to use crbegin() to iterate over the multimap in reverse order. It iterates until it reaches crend().
#include <iostream> #include <map> int main() { std::multimap<int, std::string> a = {{2, "TP"}, {3, "TutorialsPoint"}, {1, "Tutorix"}}; for (auto x = a.crbegin(); x != a.crend(); ++x) { std::cout << "(" << x->first << ", " << x->second << ")\n"; } return 0; }
Output
Following is the output of the above code −
(3, TutorialsPoint) (2, TP) (1, Tutorix)
Example
In the following example, we are going to find the specific element in reverse order.
#include <iostream> #include <map> int main() { std::multimap<int, std::string> a = {{3, "BMW"}, {1, "Cruze"}, {2, "Sail"}}; for (auto x = a.crbegin(); x != a.crend(); ++x) { if (x->first == 1) { std::cout << "" << x->second << std::endl; break; } } return 0; }
Output
Let us compile and run the above program, this will produce the following result −
Cruze
Example
Following is the example, where we are going to compare the values in the reverse order.
#include <iostream> #include <map> int main() { std::multimap<int, std::string> x = { {1, "A"}, {2, "B"}, {3, "C"} }; std::multimap<int, std::string> y = { {3, "C"}, {2, "B"}, {1, "A"} }; bool equal = std::equal(x.crbegin(), x.crend(), y.crbegin(), y.crend()); std::cout << (equal ? "Multimaps are equal." : "Multimaps are not equal.") << '\n'; return 0; }
Output
If we run the above code it will generate the following output −
Multimaps are equal.