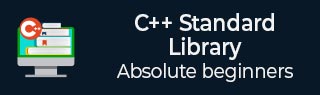
- The C Standard Library
- The C Standard Library
- The C++ Standard Library
- C++ Library - Home
- C++ Library - <fstream>
- C++ Library - <iomanip>
- C++ Library - <ios>
- C++ Library - <iosfwd>
- C++ Library - <iostream>
- C++ Library - <istream>
- C++ Library - <ostream>
- C++ Library - <sstream>
- C++ Library - <streambuf>
- C++ Library - <atomic>
- C++ Library - <complex>
- C++ Library - <exception>
- C++ Library - <functional>
- C++ Library - <limits>
- C++ Library - <locale>
- C++ Library - <memory>
- C++ Library - <new>
- C++ Library - <numeric>
- C++ Library - <regex>
- C++ Library - <stdexcept>
- C++ Library - <string>
- C++ Library - <thread>
- C++ Library - <tuple>
- C++ Library - <typeinfo>
- C++ Library - <utility>
- C++ Library - <valarray>
- The C++ STL Library
- C++ Library - <array>
- C++ Library - <bitset>
- C++ Library - <deque>
- C++ Library - <forward_list>
- C++ Library - <list>
- C++ Library - <map>
- C++ Library - <multimap>
- C++ Library - <queue>
- C++ Library - <priority_queue>
- C++ Library - <set>
- C++ Library - <stack>
- C++ Library - <unordered_map>
- C++ Library - <unordered_set>
- C++ Library - <vector>
- C++ Library - <algorithm>
- C++ Library - <iterator>
- C++ Programming Resources
- C++ Programming Tutorial
- C++ Useful Resources
- C++ Discussion
C++ multimap::clear() Function
The C++ std::multimap::clear() function is used to remove all the elements from the multimap, leaving it with a size of 0. This function ensures that the multimap size become zero, but does not dellocate the memory allocated by the multimap. It is efficient for resetting the container for reuse without destroying it. The time complexity of this function is linear i.e.O(n).
Syntax
Following is the syntax for std::multimap::clear() function.
void clear();
Parameters
This function does not accept any parameter.
Return value
This function does not return anything.
Example
Let's look at the following example, where we are going to demonstrate the usage of clear() function
#include <iostream> #include <map> int main() { std::multimap<int, std::string> x; x.insert({1, "Hi"}); x.insert({2, "Hello"}); std::cout << "Size before clear: " << x.size() << std::endl; x.clear(); std::cout << "Size after clear: " << x.size() << std::endl; return 0; }
Output
Output of the above code is as follows −
Size before clear: 2 Size after clear: 0
Example
Consider the following example, where we are going to reuse the multimap after calling clear() function.
#include <iostream> #include <map> int main() { std::multimap<int, std::string> a; a.insert({1, "Audi"}); a.clear(); a.insert({2, "Cruze"}); for (const auto& pair : a) { std::cout << pair.first << ": " << pair.second << std::endl; } return 0; }
Output
Following is the output of the above code −
2: Cruze
Example
In the following example, we are going to check, whether the multimap is empty or not before and after the clear() function.
#include <iostream> #include <map> int main() { std::multimap<int, std::string> a; a.insert({1, "TP"}); a.insert({2, "TutorialsPoint"}); std::cout << "Empty before clear : " << std::boolalpha << a.empty() << std::endl; a.clear(); std::cout << "Empty after clear : " << std::boolalpha << a.empty() << std::endl; return 0; }
Output
If we run the above code it will generate the following output −
Empty before clear : false Empty after clear : true