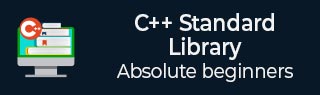
- The C Standard Library
- The C Standard Library
- The C++ Standard Library
- C++ Library - Home
- C++ Library - <fstream>
- C++ Library - <iomanip>
- C++ Library - <ios>
- C++ Library - <iosfwd>
- C++ Library - <iostream>
- C++ Library - <istream>
- C++ Library - <ostream>
- C++ Library - <sstream>
- C++ Library - <streambuf>
- C++ Library - <atomic>
- C++ Library - <complex>
- C++ Library - <exception>
- C++ Library - <functional>
- C++ Library - <limits>
- C++ Library - <locale>
- C++ Library - <memory>
- C++ Library - <new>
- C++ Library - <numeric>
- C++ Library - <regex>
- C++ Library - <stdexcept>
- C++ Library - <string>
- C++ Library - <thread>
- C++ Library - <tuple>
- C++ Library - <typeinfo>
- C++ Library - <utility>
- C++ Library - <valarray>
- The C++ STL Library
- C++ Library - <array>
- C++ Library - <bitset>
- C++ Library - <deque>
- C++ Library - <forward_list>
- C++ Library - <list>
- C++ Library - <map>
- C++ Library - <multimap>
- C++ Library - <queue>
- C++ Library - <priority_queue>
- C++ Library - <set>
- C++ Library - <stack>
- C++ Library - <unordered_map>
- C++ Library - <unordered_set>
- C++ Library - <vector>
- C++ Library - <algorithm>
- C++ Library - <iterator>
- C++ Programming Resources
- C++ Programming Tutorial
- C++ Useful Resources
- C++ Discussion
C++ multimap::cend() Function
The C++ std::multimap::cend() function is used to return a constant iterator to the element following the last element of the multimap. This iterator is used for read-only access, ensuring that the elements are not modified. It is often utilized in conjunction with cbegin() to iterate through the multimap from start to end in a read-only manner. The time complexity of this function is constant i.e.O(1).
Syntax
Following is the syntax for std::multimap::cend() function.
const_iterator cend() const noexcept;
Parameters
This function does not accept any parameter
Return value
This function returns a constant iterator pointing to the last element of the multimap.
Example
Let's look at the following example, where we are going to demonstrate the usage of cend() function.
#include <iostream> #include <map> int main() { std::multimap<int, std::string> x = {{1, "Hi"}, {2, "Hello"}, {2, "Namaste"}, {3, "Vanakam"}}; for (auto a = x.cbegin(); a != x.cend(); ++a) { std::cout << a->first << ": " << a->second << std::endl; } return 0; }
Output
Output of the above code is as follows −
1: Hi 2: Hello 2: Namaste 3: Vanakam
Example
Consider the another scenario, where we are going to perform the reverse iteration using cend() function.
#include <iostream> #include <map> int main() { std::multimap<int, std::string> a = {{1, "Tutorix"}, {2, "TP"}, {2, "TutorialsPoint"}, {3, "Welcome"}}; for (auto x = std::make_reverse_iterator(a.cend()); x != std::make_reverse_iterator(a.cbegin()); ++x) { std::cout << x->first << ": " << x->second << std::endl; } return 0; }
Output
Following is the output of the above code −
3: Welcome 2: TutorialsPoint 2: TP 1: Tutorix
Example
In the following example, we are going to find all the elements with a specific key and iterate over that range using cend() function.
#include <iostream> #include <map> int main() { std::multimap<int, std::string> x = {{1, "Audi"}, {2, "Cruze"}, {2, "Sail"}, {3, "BMW"}}; auto a = x.equal_range(2); for (auto b = a.first; b != a.second && b != x.cend(); ++b) { std::cout << b->first << ": " << b->second << std::endl; } return 0; }
Output
If we run the above code it will generate the following output −
2: Cruze 2: Sail