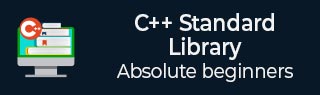
- The C Standard Library
- The C Standard Library
- The C++ Standard Library
- C++ Library - Home
- C++ Library - <fstream>
- C++ Library - <iomanip>
- C++ Library - <ios>
- C++ Library - <iosfwd>
- C++ Library - <iostream>
- C++ Library - <istream>
- C++ Library - <ostream>
- C++ Library - <sstream>
- C++ Library - <streambuf>
- C++ Library - <atomic>
- C++ Library - <complex>
- C++ Library - <exception>
- C++ Library - <functional>
- C++ Library - <limits>
- C++ Library - <locale>
- C++ Library - <memory>
- C++ Library - <new>
- C++ Library - <numeric>
- C++ Library - <regex>
- C++ Library - <stdexcept>
- C++ Library - <string>
- C++ Library - <thread>
- C++ Library - <tuple>
- C++ Library - <typeinfo>
- C++ Library - <utility>
- C++ Library - <valarray>
- The C++ STL Library
- C++ Library - <array>
- C++ Library - <bitset>
- C++ Library - <deque>
- C++ Library - <forward_list>
- C++ Library - <list>
- C++ Library - <map>
- C++ Library - <multimap>
- C++ Library - <queue>
- C++ Library - <priority_queue>
- C++ Library - <set>
- C++ Library - <stack>
- C++ Library - <unordered_map>
- C++ Library - <unordered_set>
- C++ Library - <vector>
- C++ Library - <algorithm>
- C++ Library - <iterator>
- C++ Programming Resources
- C++ Programming Tutorial
- C++ Useful Resources
- C++ Discussion
C++ multimap::begin() Function
The C++ std::multimap::begin() function is a standard container that stores key value pairs allowing multiple entries with the same key. It is used to return the iterator pointing to the first element in the container. If the multimap is empty, begin() function returns the same iterator as end(). The time complexity of this funcion is constant i.e.O(1).
The begin() function is used in conjuction with other iterators to access or manipulate elements in the multimap.
Syntax
Following is the syntax for std::multimap::begin() function.
iterator begin(); const_iterator begin() const;
Parameters
This function does not accept any parameters
Return value
This function returns an iterator pointing to the first element.
Example
Let's look at the following example, where we are going to demonstrate the usage of begin() function.
#include <iostream> #include <map> int main() { std::multimap<int, std::string> a = {{1, "TutorialsPoint"}, {2, "TP"}, {3, "Tutorix"}}; auto it = a.begin(); std::cout << it->first << ": " << it->second << std::endl; return 0; }
Output
Output of the above code is as follows −
1: TutorialsPoint
Example
Consider the following example, where we are going to use the for loop to iterate through all elements in the multimap from element pointed by begin() and ending at end().
#include <iostream> #include <map> int main() { std::multimap<int, std::string> a = {{1, "Hello"}, {2, "Namaste"}, {1, "Vanakam"}}; for(auto it = a.begin(); it != a.end(); ++it) { std::cout << it->first << ": " << it->second << std::endl; } return 0; }
Output
Following is the output of the above code −
1: Hello 1: Vanakam 2: Namaste
Example
In the following example, we are going to modify the value of the first element in the multimap using the iterator obtained by begin().
#include <iostream> #include <map> int main() { std::multimap<int, std::string> a = {{1, "Benz"}, {2, "Audi"}, {3, "Ciaz"}}; auto it = a.begin(); it->second = "Cruze"; for(const auto& p : a) { std::cout << p.first << ": " << p.second << std::endl; } return 0; }
Output
Let us compile and run the above program, this will produce the following result −
1: Cruze 2: Audi 3: Ciaz
Example
Following is the example, where we are going to check whether the multimap is empty or not.
#include <iostream> #include <map> int main() { std::multimap<int, std::string> a; if(a.begin() == a.end()) { std::cout << "Multimap is empty." << std::endl; } else { std::cout << "Multimap is not empty." << std::endl; } return 0; }
Output
If we run the above code it will generate the following output −
Multimap is empty.