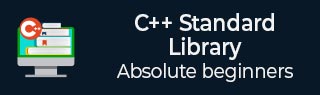
- The C Standard Library
- The C Standard Library
- The C++ Standard Library
- C++ Library - Home
- C++ Library - <fstream>
- C++ Library - <iomanip>
- C++ Library - <ios>
- C++ Library - <iosfwd>
- C++ Library - <iostream>
- C++ Library - <istream>
- C++ Library - <ostream>
- C++ Library - <sstream>
- C++ Library - <streambuf>
- C++ Library - <atomic>
- C++ Library - <complex>
- C++ Library - <exception>
- C++ Library - <functional>
- C++ Library - <limits>
- C++ Library - <locale>
- C++ Library - <memory>
- C++ Library - <new>
- C++ Library - <numeric>
- C++ Library - <regex>
- C++ Library - <stdexcept>
- C++ Library - <string>
- C++ Library - <thread>
- C++ Library - <tuple>
- C++ Library - <typeinfo>
- C++ Library - <utility>
- C++ Library - <valarray>
- The C++ STL Library
- C++ Library - <array>
- C++ Library - <bitset>
- C++ Library - <deque>
- C++ Library - <forward_list>
- C++ Library - <list>
- C++ Library - <map>
- C++ Library - <multimap>
- C++ Library - <queue>
- C++ Library - <priority_queue>
- C++ Library - <set>
- C++ Library - <stack>
- C++ Library - <unordered_map>
- C++ Library - <unordered_set>
- C++ Library - <vector>
- C++ Library - <algorithm>
- C++ Library - <iterator>
- C++ Programming Resources
- C++ Programming Tutorial
- C++ Useful Resources
- C++ Discussion
C++ multimap::insert() Function
The C++ std::multimap::insert() function is used to add elements to a multimap container. It inserts a key-value pair into the multimap, maintaining sorted order based on keys. If the key already exists, it does not overwrite the existing value, preserving the multimap unique key property. This function returns an iterator pointing to the inserted element, facilitating further operations.
This function has 4 polymorphic variants: inserting with single element, with hint, range and initializer list (you can find the syntaxes of all the variants below).
Syntax
Following is the syntax for std::multimap::insert() function.
iterator insert (const value_type& val); or iterator insert (const_iterator position, const value_type& val); or void insert (InputIterator first, InputIterator last); or void insert (initializer_list<value_type> il);
Parameters
- val − It indicates the value to be inserted.
- position − It indicates the hint for the position to insert element.
- first, last − Iterators specifying the range of the elements.
- il − It indicates the initializer list object.
Return value
This function returns an iterator pointing to the newly inserted element.
Example
Let's look at the following example, where we are going to insert the single key value pair.
#include <iostream> #include <map> int main() { std::multimap<int, std::string> a; a.insert(std::make_pair(1, "A")); a.insert(std::make_pair(2, "B")); for (const auto& pair : a) { std::cout << pair.first << ": " << pair.second << std::endl; } return 0; }
Output
Output of the above code is as follows −
1: A 2: B
Example
Consider the following example, where we are going to use the hint version and inserting an element.
#include <iostream> #include <map> int main() { std::multimap<int, std::string> a; auto x = a.begin(); x = a.insert(x, std::make_pair(1, "TutorialsPoint")); a.insert(x, std::make_pair(2, "TP")); for (const auto& pair : a) { std::cout << pair.first << ": " << pair.second << std::endl; } return 0; }
Output
Following is the output of the above code −
1: TutorialsPoint 2: TP
Example
In the following example, we are going to use the range version and inserting an element.
#include <iostream> #include <map> #include <vector> int main() { std::multimap<int, std::string> a; std::vector<std::pair<int, std::string>> x = {{1, "AB"}, {2, "BC"}, {2, "CD"}}; a.insert(x.begin(), x.end()); for (const auto& pair : a) { std::cout << pair.first << ": " << pair.second << std::endl; } return 0; }
Output
If we run the above code it will generate the following output −
1: AB 2: BC 2: CD
Example
Following is the example, where we are going to use the emplace() function with insert().
#include <iostream> #include <map> int main() { std::multimap<int, std::string> a; a.insert(std::pair<int, std::string>(2, "HI")); a.emplace(1, "HELLO"); for (const auto& pair : a) { std::cout << pair.first << ": " << pair.second << std::endl; } return 0; }
Output
Let us compile and run the above program, this will produce the following result −
1: HELLO 2: HI